用tarojs实现一个公用富文本编辑器
时间: 2024-05-03 08:23:20 浏览: 247
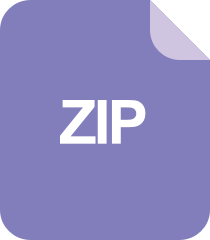
JS实现富文本编辑器
Taro 是一个开放式跨端跨框架解决方案,可以通过一套代码构建多个平台的应用,包括微信小程序、支付宝小程序、H5 等。要实现一个公用富文本编辑器,我们可以使用 Taro 编写一个小程序组件,并将其打包成可复用的 NPM 模块。以下是实现过程的大致步骤:
1. 安装 Taro CLI 工具
使用 npm 或者 yarn 全局安装 Taro CLI 工具:
```bash
npm install -g @tarojs/cli
# 或者
yarn global add @tarojs/cli
```
2. 创建 Taro 组件项目
使用 Taro CLI 工具创建一个 Taro 组件项目:
```bash
taro init --type component my-editor
```
这个命令会使用默认的模板创建一个 Taro 组件项目,包括一些示例代码,可以在其中添加我们的富文本编辑器组件。
3. 安装富文本编辑器库
我们可以使用一些现成的富文本编辑器库,如 [wangeditor](https://www.wangeditor.com/) 或 [quill](https://quilljs.com/)。这里以 wangeditor 为例:
```bash
npm install --save wangeditor
# 或者
yarn add wangeditor
```
4. 实现组件代码
在 `src/components` 目录下创建一个 `Editor` 组件,并在其中引入 wangeditor 库:
```jsx
import Taro, { Component } from '@tarojs/taro'
import { View } from '@tarojs/components'
import WangEditor from 'wangeditor'
class Editor extends Component {
componentDidMount() {
const editor = new WangEditor(this.refs.editor)
editor.create()
}
render() {
return (
<View ref="editor" style={{ height: '500px' }}>
{/* 空标签,用于挂载富文本编辑器 */}
</View>
)
}
}
export default Editor
```
在 `componentDidMount` 生命周期中,创建一个 wangeditor 实例,并将其挂载到组件的 DOM 元素上。在 `render` 方法中,使用 `View` 组件创建一个空的 DOM 元素,并将其设置为富文本编辑器的挂载点。
5. 导出组件
在 `src/index.js` 文件中,导出 `Editor` 组件:
```jsx
import Editor from './components/Editor'
export default Editor
```
6. 打包组件
使用 Taro CLI 工具打包组件:
```bash
taro build --type npm --watch
```
这个命令会将组件打包成一个 NPM 模块,并将其输出到 `dist` 目录下。
7. 使用组件
在其他 Taro 项目中,通过 npm 安装我们刚才打包的富文本编辑器组件:
```bash
npm install --save my-editor
# 或者
yarn add my-editor
```
在页面中使用 `my-editor` 组件:
```jsx
import Taro, { Component } from '@tarojs/taro'
import { View } from '@tarojs/components'
import Editor from 'my-editor'
class MyPage extends Component {
render() {
return (
<View>
<Editor />
</View>
)
}
}
export default MyPage
```
至此,我们已经完成了一个基于 Taro 的公用富文本编辑器组件的实现。
阅读全文
相关推荐
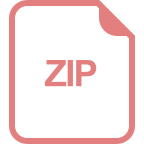
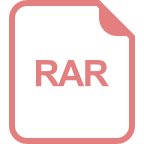
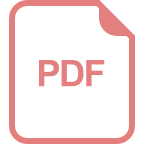
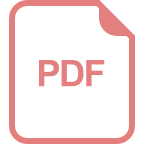
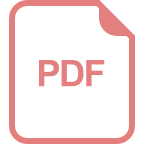
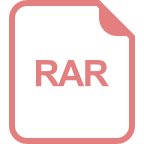
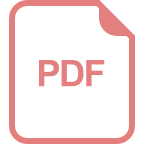
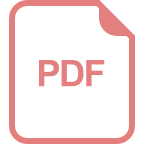
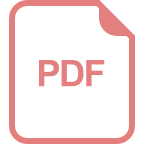
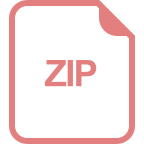
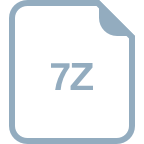
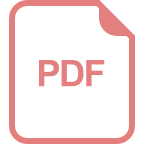
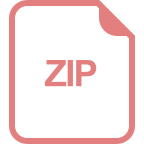
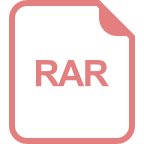