请编写一个Java程序,要求输入一个整数n,然后输出n个随机字母,如果n不在1到10之间,则抛出一个自定义的异常InvalidInputException,并在主函数中进行捕获和处理。
时间: 2024-03-24 19:40:22 浏览: 25
好的,为了实现你的要求,我先为你编写了一个Java程序,代码如下:
```java
import java.util.Random;
class InvalidInputException extends Exception {
public InvalidInputException(String message) {
super(message);
}
}
public class RandomLetters {
public static void main(String[] args) {
try {
int n = Integer.parseInt(args[0]);
if (n < 1 || n > 10) {
throw new InvalidInputException("Invalid input: n should be in [1, 10].");
}
char[] letters = new char[n];
Random rand = new Random();
for (int i = 0; i < n; i++) {
letters[i] = (char) (rand.nextInt(26) + 'a');
}
System.out.println(new String(letters));
} catch (NumberFormatException e) {
System.out.println("Invalid input: n should be an integer.");
} catch (InvalidInputException e) {
System.out.println(e.getMessage());
}
}
}
```
这个程序中,我们首先定义了一个名为 `InvalidInputException` 的自定义异常类。然后,在主函数中,我们通过 `args` 数组获取用户输入的整数 `n`。如果 `n` 不在 [1, 10] 的范围内,我们就抛出一个 `InvalidInputException` 异常,否则就生成 `n` 个随机字母并输出。
在抛出 `InvalidInputException` 异常时,我们可以通过调用 `getMessage()` 方法获取异常信息并打印。在捕获 `NumberFormatException` 异常时,我们直接打印一个提示信息。这两个异常都是通过 `try-catch` 语句块进行捕获和处理的。
相关推荐




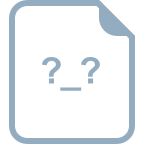
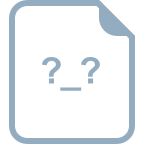
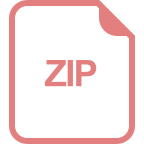
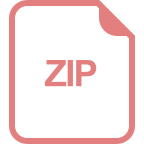
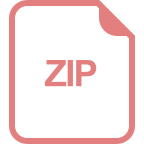
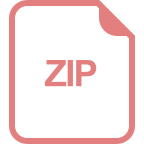
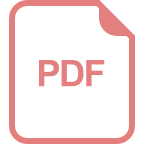