用户输入食材,程序匹配相应的菜谱,供用户选择。请根据上面这段话构思一个程序的主干。如何运用c++面向对象语言实现匹配相应菜谱
时间: 2023-07-15 08:15:21 浏览: 164
程序主干如下:
1. 用户输入食材
2. 程序根据输入的食材进行菜谱匹配
3. 程序输出相应的菜谱供用户选择
要实现匹配相应菜谱的功能,可以考虑使用面向对象编程思想。可以定义一个菜谱类,其中包含菜名、所需食材等属性和方法。程序可以先读取一个菜谱库,将所有菜谱存储在一个数组或容器中。
当用户输入食材时,程序可以遍历所有菜谱,查找包含输入食材的菜谱,并将这些菜谱输出给用户。可以定义一个函数来完成这个任务,函数的输入参数是用户输入的食材,输出参数是匹配到的菜谱列表。
可以通过如下代码实现:
```c++
#include <iostream>
#include <vector>
using namespace std;
// 菜谱类
class Recipe {
private:
string name; // 菜名
vector<string> ingredients; // 所需食材
public:
Recipe(string name, vector<string> ingredients) {
this->name = name;
this->ingredients = ingredients;
}
string getName() {
return name;
}
vector<string> getIngredients() {
return ingredients;
}
};
// 菜谱库
vector<Recipe> recipeList = {
Recipe("青椒炒肉", {"青椒", "猪肉"}),
Recipe("番茄炒蛋", {"番茄", "鸡蛋"}),
Recipe("西红柿牛肉面", {"西红柿", "牛肉", "面条"})
};
// 查找包含指定食材的菜谱
vector<Recipe> findRecipeByIngredient(string ingredient) {
vector<Recipe> result;
for (Recipe recipe : recipeList) {
vector<string> ingredients = recipe.getIngredients();
for (string item : ingredients) {
if (item == ingredient) {
result.push_back(recipe);
break; // 跳出内层循环
}
}
}
return result;
}
int main() {
string input;
cout << "请输入食材:";
cin >> input;
vector<Recipe> matchedRecipes = findRecipeByIngredient(input);
if (matchedRecipes.size() == 0) {
cout << "没有找到相应的菜谱" << endl;
} else {
cout << "找到以下菜谱:" << endl;
for (Recipe recipe : matchedRecipes) {
cout << recipe.getName() << endl;
}
}
return 0;
}
```
这个程序首先定义了一个 Recipe 类,表示菜谱。其属性包括菜名和所需食材。程序还定义了一个 recipeList 容器,存储所有菜谱。
程序的核心部分是 findRecipeByIngredient 函数,它遍历所有菜谱,查找包含指定食材的菜谱,并将这些菜谱存储在一个 vector 容器中返回。
最后,程序读入用户输入的食材,调用 findRecipeByIngredient 函数,输出匹配到的菜谱列表。如果没有匹配到任何菜谱,则输出 "没有找到相应的菜谱"。
阅读全文
相关推荐
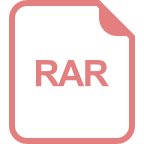
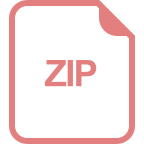
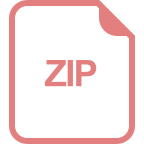
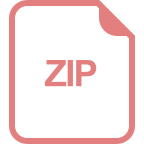
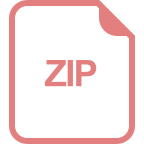
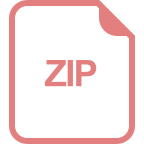
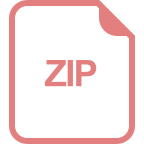
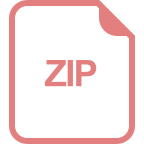
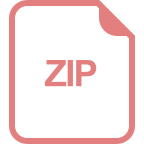
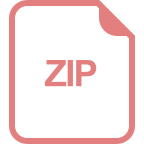
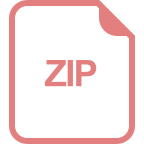
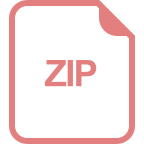
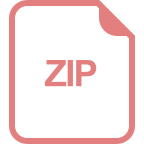
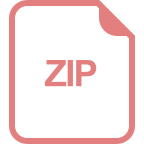
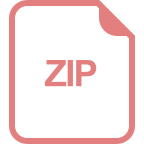
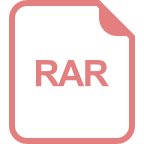