vue 实现数据滚动显示_vue表格数据打印功能的实现(打印全部数据)
时间: 2024-03-23 22:42:28 浏览: 13
实现数据滚动显示:
1. 在Vue组件中定义一个滚动容器,设置好宽度和高度,overflow属性设置为auto
2. 在滚动容器内部定义一个表格,将表格的宽度设置为100%,这样表格就会充满整个滚动容器
3. 使用v-for指令循环输出表格数据,同时根据需求决定每次输出的数据数量,当数据量超过容器高度时就会出现滚动条
代码示例:
```html
<template>
<div class="scroll-container">
<table>
<thead>
<tr>
<th>编号</th>
<th>姓名</th>
<th>年龄</th>
<th>地址</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in tableData" :key="index">
<td>{{ item.id }}</td>
<td>{{ item.name }}</td>
<td>{{ item.age }}</td>
<td>{{ item.address }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<style>
.scroll-container {
width: 400px;
height: 300px;
overflow: auto;
}
table {
width: 100%;
}
</style>
```
实现表格数据打印:
1. 在Vue组件中定义一个按钮,绑定打印函数
2. 在打印函数中,通过JavaScript获取到表格元素,然后创建一个新的窗口,将表格元素添加到新窗口中,然后调用窗口的打印函数即可实现打印
代码示例:
```html
<template>
<div>
<div class="scroll-container">
<table>
<thead>
<tr>
<th>编号</th>
<th>姓名</th>
<th>年龄</th>
<th>地址</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in tableData" :key="index">
<td>{{ item.id }}</td>
<td>{{ item.name }}</td>
<td>{{ item.age }}</td>
<td>{{ item.address }}</td>
</tr>
</tbody>
</table>
</div>
<button @click="printTable">打印</button>
</div>
</template>
<script>
export default {
data() {
return {
tableData: [
{ id: 1, name: '张三', age: 20, address: '北京市' },
{ id: 2, name: '李四', age: 22, address: '上海市' },
{ id: 3, name: '王五', age: 25, address: '广州市' },
{ id: 4, name: '赵六', age: 28, address: '深圳市' },
{ id: 5, name: '田七', age: 32, address: '杭州市' },
{ id: 6, name: '刘八', age: 35, address: '成都市' },
{ id: 7, name: '孙九', age: 40, address: '重庆市' },
{ id: 8, name: '周十', age: 45, address: '武汉市' }
]
}
},
methods: {
printTable() {
const table = document.querySelector('table')
const newWindow = window.open('', '_blank')
newWindow.document.write(`<html><head><title>表格打印</title></head><body>${table.outerHTML}</body></html>`)
newWindow.print()
newWindow.close()
}
}
}
</script>
<style>
.scroll-container {
width: 400px;
height: 300px;
overflow: auto;
}
table {
width: 100%;
}
</style>
```
相关推荐
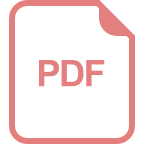
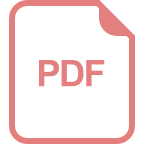
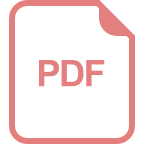
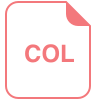
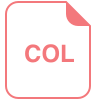












