Connection conn=null; PreparedStatement ps = null; try { String url = "jdbc:mysql://127.0.0.1:3306/tsgc?useUnicode=true&characterEncoding=utf8"; String user = "root"; String password = "123123"; conn = DriverManager.getConnection(url, user, password); // 第三步:建立statement对象 String sql="update employee set password='hello' where sex='女'"; ps=conn.prepareStatement(sql); // 第四步:修改数据 ps.execute(); // 第五步:关闭statement对象和连接对象 }catch(SQLException e){ e.printStackTrace(); }finally{ try{ ps.close(); conn.close(); }catch(SQLException e){ e.printStackTrace(); } } 逐行解释
时间: 2024-02-15 15:28:19 浏览: 87
这段代码是使用Java程序连接到MySQL数据库,并执行一条SQL语句更新数据库中的数据。下面是逐行解释:
1. `Connection conn=null;` :定义一个变量conn,用于存储连接对象。
2. `PreparedStatement ps = null;` :定义一个变量ps,用于存储PreparedStatement对象。
3. `try {` :使用try语句处理可能出现的异常。
4. `String url = "jdbc:mysql://127.0.0.1:3306/tsgc?useUnicode=true&characterEncoding=utf8";` :定义一个字符串url,存储MySQL数据库的URL地址。
5. `String user = "root";` :定义一个字符串user,存储MySQL数据库的用户名。
6. `String password = "123123";` :定义一个字符串password,存储MySQL数据库的密码。
7. `conn = DriverManager.getConnection(url, user, password);` :使用DriverManager类的静态方法getConnection()连接到MySQL数据库,并将连接对象赋值给变量conn。
8. `String sql="update employee set password='hello' where sex='女'";` :定义一个字符串sql,存储要执行的SQL语句。
9. `ps=conn.prepareStatement(sql);` :使用连接对象conn的prepareStatement()方法创建一个PreparedStatement对象,并将其赋值给变量ps。
10. `ps.execute();` :使用PreparedStatement对象ps的execute()方法执行SQL语句,更新数据库中的数据。
11. `}catch(SQLException e){` :如果出现SQLException异常,则执行catch语句块中的代码。
12. `e.printStackTrace();` :在控制台输出异常信息,以便开发人员进行调试和排错。
13. `}finally{` :无论try语句块中是否出现异常,都会执行finally语句块中的代码。
14. `ps.close();` :使用PreparedStatement对象ps的close()方法关闭对象。
15. `conn.close();` :使用连接对象conn的close()方法关闭连接。
16. `}catch(SQLException e){` :如果出现SQLException异常,则执行catch语句块中的代码。
17. `e.printStackTrace();` :在控制台输出异常信息,以便开发人员进行调试和排错。
总的来说,这段代码的作用就是连接到MySQL数据库,并执行一条SQL语句更新数据库中的数据。最后,关闭连接和PreparedStatement对象。
阅读全文
相关推荐





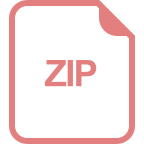



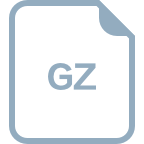



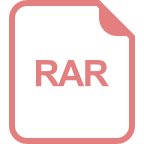




