帮我写一下使用Python将word和pdf格式的文件批量转为图片的代码
时间: 2023-06-26 11:04:41 浏览: 60
好的,可以使用Python的`docx2pdf`和`wand`这两个库来实现将Word和PDF文件批量转为图片的功能。你需要先安装这两个库,可以使用以下命令:
```
pip install docx2pdf wand
```
接下来是代码实现:
```python
from docx2pdf import convert
from wand.image import Image
# Word 转 PDF
convert("input.docx") # 将 input.docx 转为 input.pdf
# PDF 转图片
with Image(filename="input.pdf") as img:
with img.convert('png') as converted:
converted.save(filename='output.png')
```
上面的代码中,首先使用`docx2pdf`库将Word文件转为PDF格式,然后使用`wand`库将PDF文件转为PNG格式的图片,并保存为`output.png`。
如果你要批量转换文件,可以使用`os`库来遍历文件夹,将上面的代码封装为一个函数,如下所示:
```python
import os
from docx2pdf import convert
from wand.image import Image
def convert_to_image(file_path):
filename, ext = os.path.splitext(file_path)
if ext == ".docx":
# Word 转 PDF
convert(file_path)
pdf_path = filename + ".pdf"
elif ext == ".pdf":
pdf_path = file_path
else:
return # 不是 Word 或 PDF 文件,跳过处理
# PDF 转图片
with Image(filename=pdf_path) as img:
with img.convert('png') as converted:
output_path = filename + ".png"
converted.save(filename=output_path)
# 遍历文件夹
folder_path = "/path/to/folder"
for root, dirs, files in os.walk(folder_path):
for file in files:
file_path = os.path.join(root, file)
convert_to_image(file_path)
```
上面的代码将`convert_to_image`函数封装为一个批量处理的函数,可以遍历指定的文件夹,将所有的Word和PDF文件转为PNG格式的图片,并保存在同一目录下。
相关推荐
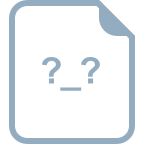
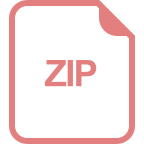














