用JAVA编写俄罗斯方块游戏android
时间: 2024-04-28 17:19:53 浏览: 177
好的,编写俄罗斯方块游戏的第一步是了解游戏的规则和逻辑。俄罗斯方块游戏的规则是:玩家控制下落的方块,使其在底部堆积成一行或多行,当堆积的方块触及顶部时,游戏结束。游戏中的方块由四个小方块组成,每个小方块可以是七种不同的颜色之一。
接下来,我们可以使用Java语言编写俄罗斯方块游戏的核心逻辑。我们需要创建一个方块类来表示游戏中的方块,这个类应该包含方块的位置、颜色、形状等属性。然后,我们需要创建一个游戏类来管理游戏的状态、计分等信息,这个类应该包含游戏界面、方块的下落速度、当前下落的方块等属性。
接下来,我们可以使用Android Studio创建一个新的Android应用程序,并在应用程序中添加俄罗斯方块游戏的UI组件,例如游戏界面、得分板等。然后,我们可以将我们编写的Java代码与UI组件集成起来,实现俄罗斯方块游戏的功能。
最后,我们需要对游戏进行测试,并进行必要的调试和优化。我们可以使用Android模拟器或实际设备进行测试,确保游戏在不同的设备上都能正常运行。
以上是一个简单的流程,希望能对您有所帮助。
相关问题
利用Java语言,使用Android studio 软件进行编写一个俄罗斯方块小游戏,生成代码
很好的问题!编写俄罗斯方块小游戏是Java语言和Android Studio的一个不错的练习。以下是一些基本步骤,以帮助您开始编写代码:
1. 设置开发环境:您需要安装Java JDK和Android Studio软件,以及设置开发环境。
2. 创建新的Android Studio项目:打开Android Studio软件,点击“新建项目”,选择“空活动”,并输入应用程序名称和包名称。
3. 设计游戏UI:使用Android Studio的设计工具,您可以创建游戏UI,并将其保存到res/layout文件夹中。
4. 编写Java代码:在Android Studio中创建Java类,并编写代码来处理游戏逻辑。您需要实现俄罗斯方块游戏的主要功能,例如旋转、移动和删除方块。
5. 运行游戏:使用Android Studio的模拟器或连接到Android设备来测试游戏。
下面是一些Java代码片段,可以帮助您开始编写俄罗斯方块游戏:
```java
public class Block {
private int[][] blockShape;
private int blockColor;
public Block(int[][] blockShape, int blockColor) {
this.blockShape = blockShape;
this.blockColor = blockColor;
}
public int[][] getBlockShape() {
return blockShape;
}
public int getBlockColor() {
return blockColor;
}
}
public class Game {
private Block currentBlock;
private int[][] gameBoard;
public Game() {
// Initialize the game board and set the current block to a random shape and color.
}
public void moveLeft() {
// Move the current block to the left on the game board.
}
public void moveRight() {
// Move the current block to the right on the game board.
}
public void rotate() {
// Rotate the current block on the game board.
}
public void drop() {
// Drop the current block down on the game board until it hits another block or the bottom of the board.
}
public void deleteRows() {
// Delete any completed rows on the game board.
}
public boolean isGameOver() {
// Check if the game is over.
}
}
```
以上是一些示例代码,但是编写完整的俄罗斯方块游戏需要更多的代码。希望这些提示可以帮助您开始编写自己的游戏!
阅读全文
相关推荐







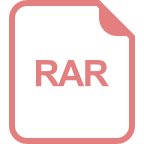









