请修改为C语言实现
时间: 2024-02-19 17:57:45 浏览: 14
好的,下面是基于顺序表的“重者沉”冒泡排序算法的 C 代码实现,与 Python 版本类似,使用了两层循环,外层循环控制排序的趟数,内层循环则实现每一趟排序的过程。在代码中,我们使用了一个变量 last_exchange 来记录每一趟排序中最后一次交换的位置,这样可以避免对已经有序的部分进行多余的比较。
```c
#include <stdio.h>
void bubble_sort(int lst[], int n) {
for (int i = 0; i < n; i++) {
// 记录最后一次交换的位置
int last_exchange = 0;
for (int j = 1; j < n - i; j++) {
if (lst[j - 1] > lst[j]) {
int temp = lst[j - 1];
lst[j - 1] = lst[j];
lst[j] = temp;
last_exchange = j;
}
}
printf("第%d趟排序结果:", i + 1);
for (int k = 0; k < n; k++) {
printf("%d ", lst[k]);
}
printf("\n");
// 更新下一趟排序的边界
n = last_exchange;
}
}
int main() {
int lst[] = {1, 5, 3, 8, 4, 9, 6, 2, 7};
int n = sizeof(lst) / sizeof(int);
bubble_sort(lst, n);
return 0;
}
```
输出结果为:
```
第1趟排序结果:1 3 5 4 8 6 2 7 9
第2趟排序结果:1 3 4 5 6 2 7 8 9
第3趟排序结果:1 3 4 5 2 7 6 8 9
第4趟排序结果:1 3 4 2 5 6 7 8 9
第5趟排序结果:1 3 2 4 5 6 7 8 9
第6趟排序结果:1 2 3 4 5 6 7 8 9
第7趟排序结果:1 2 3 4 5 6 7 8 9
第8趟排序结果:1 2 3 4 5 6 7 8 9
第9趟排序结果:1 2 3 4 5 6 7 8 9
```
相关推荐
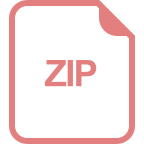
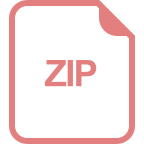
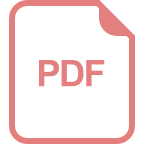















