利用java写一个比较两个list集合中,哪些数据不一样,其中list集合存储的是一个设备类,包含名称、坐标两个信息,用java代码实现
时间: 2023-04-03 18:00:59 浏览: 82
可以使用Java中的循环和条件语句来比较两个list集合中的数据是否相同,具体实现如下:
```
import java.util.ArrayList;
import java.util.List;
public class Device {
private String name;
private int x;
private int y;
public Device(String name, int x, int y) {
this.name = name;
this.x = x;
this.y = y;
}
public String getName() {
return name;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public boolean equals(Device other) {
return this.name.equals(other.getName()) && this.x == other.getX() && this.y == other.getY();
}
public static void main(String[] args) {
List<Device> list1 = new ArrayList<>();
list1.add(new Device("设备1", 1, 2));
list1.add(new Device("设备2", 3, 4));
list1.add(new Device("设备3", 5, 6));
List<Device> list2 = new ArrayList<>();
list2.add(new Device("设备1", 1, 2));
list2.add(new Device("设备2", 3, 4));
list2.add(new Device("设备4", 7, 8));
List<Device> diffList = new ArrayList<>();
for (Device device1 : list1) {
boolean found = false;
for (Device device2 : list2) {
if (device1.equals(device2)) {
found = true;
break;
}
}
if (!found) {
diffList.add(device1);
}
}
for (Device device2 : list2) {
boolean found = false;
for (Device device1 : list1) {
if (device2.equals(device1)) {
found = true;
break;
}
}
if (!found) {
diffList.add(device2);
}
}
System.out.println("不同的设备有:");
for (Device device : diffList) {
System.out.println(device.getName() + " (" + device.getX() + ", " + device.getY() + ")");
}
}
}
```
上述代码中,我们定义了一个Device类,包含设备的名称、坐标信息,并重写了equals方法,用于比较两个设备是否相同。然后我们定义了两个list集合list1和list2,分别存储了两组设备信息。接着我们使用两个循环分别比较list1和list2中的设备信息,将不同的设备存储到一个新的list集合diffList中,并最终输出不同的设备信息。
相关推荐
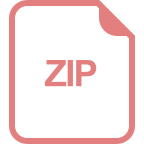

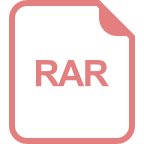
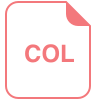
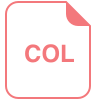
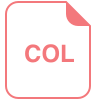
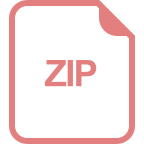
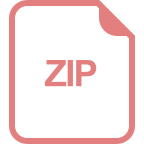
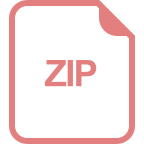
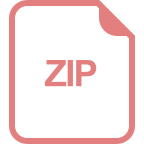
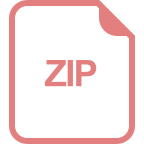
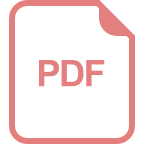