计算机图形学图形二维变换vscode实现代码
时间: 2023-07-16 07:15:58 浏览: 157
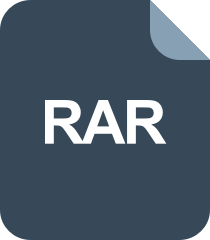
计算机图形学-二维变换与裁剪.rar
以下是使用 Python 和 Pygame 库实现图形的二维变换的示例代码:
```python
import pygame
import math
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 初始化 Pygame
pygame.init()
# 设置窗口尺寸
size = (700, 500)
screen = pygame.display.set_mode(size)
# 设置窗口标题
pygame.display.set_caption("2D Transformations")
# 设置字体
font = pygame.font.SysFont('Calibri', 25, True, False)
# 定义几何图形
triangle = [(100, 100), (150, 150), (50, 150)]
square = [(300, 100), (400, 100), (400, 200), (300, 200)]
pentagon = [(550, 150), (600, 100), (650, 125), (625, 175), (575, 175)]
# 定义变换矩阵
translation_matrix = lambda x, y: [[1, 0, x], [0, 1, y], [0, 0, 1]]
rotation_matrix = lambda theta: [[math.cos(theta), -math.sin(theta), 0], [math.sin(theta), math.cos(theta), 0], [0, 0, 1]]
scaling_matrix = lambda sx, sy: [[sx, 0, 0], [0, sy, 0], [0, 0, 1]]
# 定义变换操作
def translate(points, x, y):
matrix = translation_matrix(x, y)
return [tuple(matrix @ (p[0], p[1], 1))[:2] for p in points]
def rotate(points, theta):
matrix = rotation_matrix(theta)
return [tuple(matrix @ (p[0], p[1], 1))[:2] for p in points]
def scale(points, sx, sy):
matrix = scaling_matrix(sx, sy)
return [tuple(matrix @ (p[0], p[1], 1))[:2] for p in points]
# 定义变量
angle = 0
scale_factor = 1
tx = 0
ty = 0
# 游戏循环
done = False
while not done:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
elif event.type == pygame.KEYDOWN:
# 处理按键事件
if event.key == pygame.K_LEFT:
tx -= 10
elif event.key == pygame.K_RIGHT:
tx += 10
elif event.key == pygame.K_UP:
scale_factor *= 2
elif event.key == pygame.K_DOWN:
scale_factor /= 2
elif event.key == pygame.K_SPACE:
angle += math.pi / 4
# 清屏
screen.fill(WHITE)
# 应用变换
transformed_triangle = scale(rotate(translate(triangle, tx, ty), angle), scale_factor, scale_factor)
transformed_square = scale(rotate(translate(square, tx, ty), angle), scale_factor, scale_factor)
transformed_pentagon = scale(rotate(translate(pentagon, tx, ty), angle), scale_factor, scale_factor)
# 绘制几何图形
pygame.draw.polygon(screen, RED, transformed_triangle, 2)
pygame.draw.polygon(screen, GREEN, transformed_square, 2)
pygame.draw.polygon(screen, BLUE, transformed_pentagon, 2)
# 显示变换信息
text1 = font.render("LEFT/RIGHT: Translate", True, BLACK)
text2 = font.render("UP/DOWN: Scale", True, BLACK)
text3 = font.render("SPACE: Rotate", True, BLACK)
screen.blit(text1, [10, 10])
screen.blit(text2, [10, 40])
screen.blit(text3, [10, 70])
# 更新屏幕
pygame.display.flip()
# 退出 Pygame
pygame.quit()
```
在这个示例中,我们使用了 Pygame 库来创建一个窗口,并绘制三个几何图形:三角形、正方形和五边形。我们定义了三个变换操作:平移、旋转和缩放,并使用这些操作来对几何图形进行变换。我们使用了三个变量来控制变换:平移量 `tx` 和 `ty`、缩放因子 `scale_factor` 和旋转角度 `angle`。我们在游戏循环中不断更新这些变量,并重新计算变换后的几何图形。我们还使用 Pygame 的文本渲染功能来显示变换信息。最后,我们使用 `pygame.display.flip()` 方法更新屏幕。
阅读全文
相关推荐
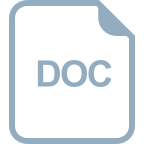
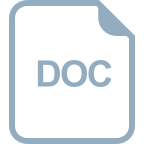
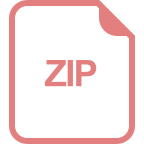
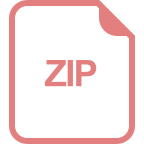
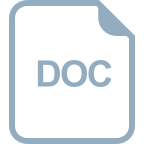
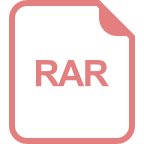
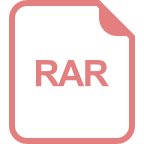
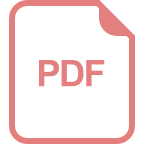
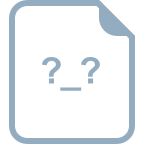
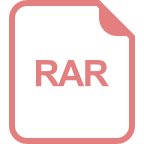
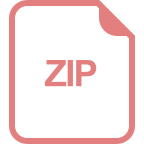
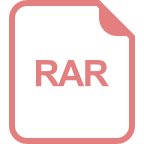
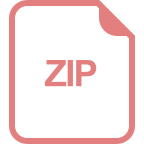
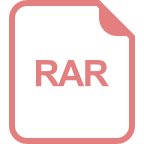
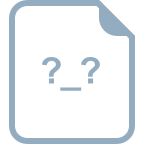
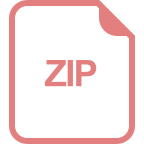
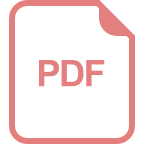
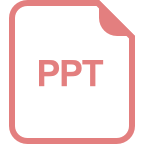