商品管理系统 要求: 1) 该系统主要处理商场中的商品信息。 2) 商品信息主要包括:商品编号、商品类型、价格、供应商等内容。 3) 供应商信息主要包括:供应商编号、供应商名称、联系电话等内容。 4) 完成以下的操作:实现商品信息、商品类型、供应商信息的添加、修改、删除和查询。 提示: 1)建立商品类、商品类型类、供应商类 2)商品、商品类型、供应商信息的初始化 3)商品信息的添加、修改、删除、查找 4)供应商信息的添加、修改、删除、查找 5)商品信息、供应商信息的输出
时间: 2023-07-10 08:33:43 浏览: 82
以下是一个简单的商品管理系统的代码示例,实现了上述要求:
```python
class Product:
def __init__(self, id, type, price, supplier):
self.id = id
self.type = type
self.price = price
self.supplier = supplier
class ProductType:
def __init__(self, id, name):
self.id = id
self.name = name
class Supplier:
def __init__(self, id, name, phone):
self.id = id
self.name = name
self.phone = phone
class ProductManager:
def __init__(self):
self.products = []
self.types = []
self.suppliers = []
def add_product(self, product):
self.products.append(product)
def update_product(self, product):
for i, p in enumerate(self.products):
if p.id == product.id:
self.products[i] = product
break
def delete_product(self, product):
self.products.remove(product)
def find_product_by_id(self, id):
for p in self.products:
if p.id == id:
return p
return None
def add_product_type(self, type):
self.types.append(type)
def update_product_type(self, type):
for i, t in enumerate(self.types):
if t.id == type.id:
self.types[i] = type
break
def delete_product_type(self, type):
self.types.remove(type)
def find_product_type_by_id(self, id):
for t in self.types:
if t.id == id:
return t
return None
def add_supplier(self, supplier):
self.suppliers.append(supplier)
def update_supplier(self, supplier):
for i, s in enumerate(self.suppliers):
if s.id == supplier.id:
self.suppliers[i] = supplier
break
def delete_supplier(self, supplier):
self.suppliers.remove(supplier)
def find_supplier_by_id(self, id):
for s in self.suppliers:
if s.id == id:
return s
return None
def print_products(self):
for p in self.products:
print("id:", p.id, "type:", p.type, "price:", p.price, "supplier:", p.supplier)
def print_suppliers(self):
for s in self.suppliers:
print("id:", s.id, "name:", s.name, "phone:", s.phone)
# 初始化商品、商品类型、供应商信息
manager = ProductManager()
t1 = ProductType(1, "衣服")
t2 = ProductType(2, "鞋子")
s1 = Supplier(1, "供应商1", "12345678")
s2 = Supplier(2, "供应商2", "87654321")
p1 = Product(1, t1, 100, s1)
p2 = Product(2, t1, 200, s2)
p3 = Product(3, t2, 300, s1)
manager.add_product_type(t1)
manager.add_product_type(t2)
manager.add_supplier(s1)
manager.add_supplier(s2)
manager.add_product(p1)
manager.add_product(p2)
manager.add_product(p3)
# 添加、修改、删除商品信息
p4 = Product(4, t2, 400, s2)
manager.add_product(p4)
p1.price = 150
manager.update_product(p1)
manager.delete_product(p2)
# 查找商品信息
p = manager.find_product_by_id(3)
if p:
print("id:", p.id, "type:", p.type.name, "price:", p.price, "supplier:", p.supplier.name)
else:
print("not found")
# 添加、修改、删除供应商信息
s3 = Supplier(3, "供应商3", "11111111")
manager.add_supplier(s3)
s2.phone = "88888888"
manager.update_supplier(s2)
manager.delete_supplier(s1)
# 输出商品信息和供应商信息
manager.print_products()
manager.print_suppliers()
```
这个示例中,我们定义了三个类:商品类(Product)、商品类型类(ProductType)和供应商类(Supplier),以及一个商品管理类(ProductManager)。商品管理类中包含了添加、修改、删除、查找商品、商品类型和供应商信息的方法,以及输出商品信息和供应商信息的方法。在初始化时,我们添加了几个商品、商品类型和供应商信息,然后进行了一些操作,最后输出了商品信息和供应商信息。
阅读全文
相关推荐

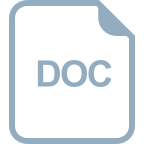
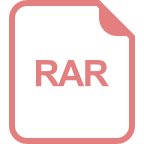
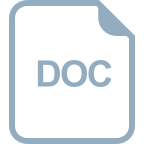
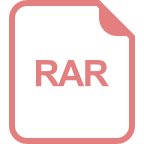
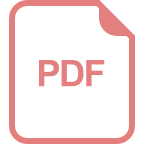
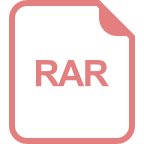
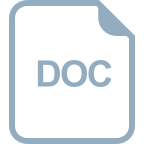
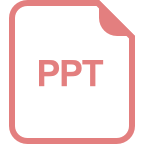
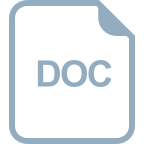
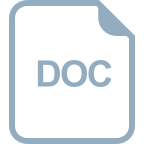
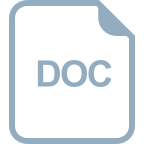
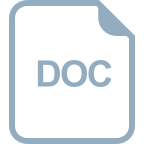
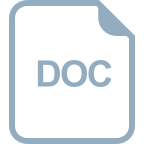
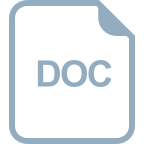