在unity中制作当游戏开始时,按下空格键产生砖墙,点击鼠标左键设计砖块。 提示: 1.利用协程或者延时方法产生砖墙 2.利用从主摄像机发射射线射击砖墙 并统计10秒内共击落多少砖块 计时10秒后跳转场景,并在新场景中分3行显示相应信息 >=30:优秀 20-29:良好 10-19:一般 <10:不合格
时间: 2024-03-24 09:41:21 浏览: 106
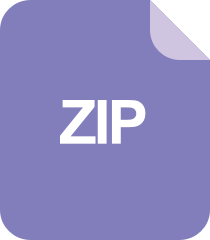
基于Unity引擎的射击游戏设计(文档+源码+开题报告).zip

好的,我会尽力回答你的问题。首先,制作这个游戏需要以下步骤:
1. 创建一个空项目,并在其中创建一个场景。
2. 在场景中创建一个平面作为地面,并将其缩放至适当大小。
3. 创建一个砖墙的预制件,并将其添加到场景中。
4. 编写脚本来实现按下空格键产生砖墙的功能。可以使用协程或延时方法来实现。
5. 编写脚本来实现点击鼠标左键设计砖块的功能。可以使用从主摄像机发射射线来实现,当射线碰撞到砖墙时,将其销毁。
6. 编写脚本来统计10秒内共击落多少砖块,并在计时10秒后跳转场景,并在新场景中分3行显示相应信息。
下面是代码示例:
```csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class GameController : MonoBehaviour
{
public GameObject brickWallPrefab; // 砖墙的预制件
public float spawnInterval = 0.5f; // 砖墙产生的间隔时间
public float gameTime = 10f; // 游戏时间
private int score = 0; // 击落的砖块数量
private float timeLeft; // 剩余游戏时间
private bool gameStarted = false; // 游戏是否已开始
// Start is called before the first frame update
void Start()
{
timeLeft = gameTime;
}
// Update is called once per frame
void Update()
{
if (Input.GetKeyDown(KeyCode.Space))
{
if (!gameStarted)
{
StartCoroutine(SpawnBrickWall());
gameStarted = true;
}
}
if (Input.GetMouseButtonDown(0))
{
RaycastHit hit;
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
if (Physics.Raycast(ray, out hit))
{
if (hit.collider.tag == "Brick")
{
Destroy(hit.collider.gameObject);
score++;
}
}
}
if (gameStarted)
{
timeLeft -= Time.deltaTime;
if (timeLeft <= 0)
{
EndGame();
}
}
}
IEnumerator SpawnBrickWall()
{
while (true)
{
Instantiate(brickWallPrefab, new Vector3(Random.Range(-5f, 5f), 0.5f, 10f), Quaternion.identity);
yield return new WaitForSeconds(spawnInterval);
}
}
void EndGame()
{
string result;
if (score >= 30)
{
result = "优秀";
}
else if (score >= 20)
{
result = "良好";
}
else if (score >= 10)
{
result = "一般";
}
else
{
result = "不合格";
}
PlayerPrefs.SetInt("Score", score);
PlayerPrefs.SetString("Result", result);
SceneManager.LoadScene("ResultScene");
}
}
```
在上面的代码中,我们使用了协程来实现砖墙的产生,并使用射线来检测砖块的击落。同时,我们还使用了PlayerPrefs来保存得分和结果,并在游戏结束后跳转到结果场景来显示信息。
希望这个回答能够对你有所帮助!
阅读全文
相关推荐
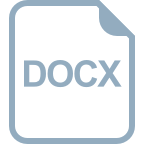
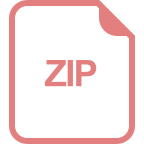
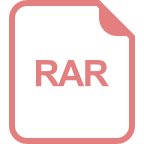
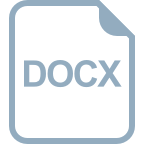
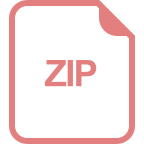
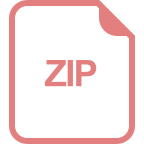
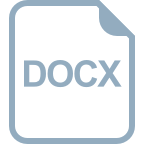
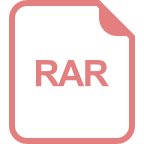
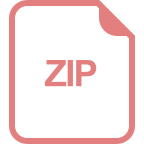
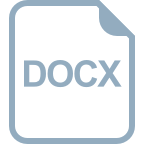
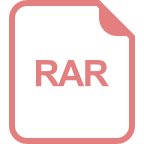
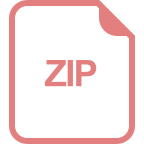
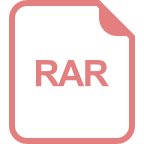
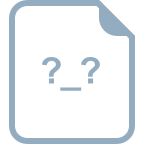
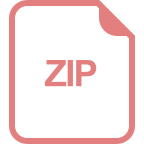
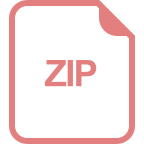