输入一行字符,最多20个,统计其中英文字母的数量,并输出C++
时间: 2023-10-06 08:05:59 浏览: 38
以下是统计英文字母数量的 C++ 代码:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str;
int count = 0;
cout << "请输入一行字符(最多20个):" << endl;
getline(cin, str);
for (int i = 0; i < str.length(); i++) {
if ((str[i] >= 'a' && str[i] <= 'z') || (str[i] >= 'A' && str[i] <= 'Z')) {
count++;
}
}
cout << "英文字母数量为:" << count << endl;
return 0;
}
```
这段代码使用了 `string` 类型的变量 `str` 来存储输入的一行字符,使用了 `getline` 函数来获取用户输入的字符串。然后使用一个 `for` 循环遍历字符串的每一个字符,判断是否是英文字母,如果是则计数器加一。最后输出计数器的值即为英文字母数量。
相关问题
用c++实现 输入一行文字,最多80个字符,统计出其中英文大写字母,小写字母,数字,空格以及其他字符的个数。 要求在主函数中输入一行文字,并输出其中各种字符的个数;在count函数中统计出这行文字中英文大写字母, 小写字母,数字,空格以及其他字符的个数。
以下是用 C++ 实现的代码:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
void count(string str) {
int upper = 0, lower = 0, digit = 0, space = 0, other = 0;
for (int i = 0; i < str.length(); i++) {
if (str[i] >= 'A' && str[i] <= 'Z') {
upper++;
} else if (str[i] >= 'a' && str[i] <= 'z') {
lower++;
} else if (str[i] >= '0' && str[i] <= '9') {
digit++;
} else if (str[i] == ' ') {
space++;
} else {
other++;
}
}
cout << "大写字母个数:" << upper << endl;
cout << "小写字母个数:" << lower << endl;
cout << "数字个数:" << digit << endl;
cout << "空格个数:" << space << endl;
cout << "其他字符个数:" << other << endl;
}
int main() {
string str;
cout << "请输入一行文字(最多80个字符):" << endl;
getline(cin, str);
count(str);
return 0;
}
```
首先在主函数中输入一行文字,使用 getline 函数可以输入包含空格的字符串。然后调用 count 函数统计各种字符的个数,遍历字符串中的每个字符,判断其属于哪种类型的字符,最后输出各种字符的个数。
使用c++编写程序,要求输入一行文字,最多80个字符,统计出其中英文大写字母,小写字母,数字,空格以及其他字符的个数。要求在主函数中输入一行文字,并输出其中各种字符的个数;在count函数中统计出这行文字中英文大写字母,小写字母,数字,空格以及其他字符的个数。
好的,这是一个比较基础的编程问题,下面是C++的代码实现:
```
#include <iostream>
using namespace std;
void count(string str);
int main() {
string str;
cout << "请输入一行文字(最多80个字符):" << endl;
getline(cin, str);
count(str);
return 0;
}
void count(string str) {
int upper = 0, lower = 0, digit = 0, space = 0, other = 0;
for (int i = 0; i < str.length(); i++) {
if (isupper(str[i])) {
upper++;
} else if (islower(str[i])) {
lower++;
} else if (isdigit(str[i])) {
digit++;
} else if (isspace(str[i])) {
space++;
} else {
other++;
}
}
cout << "大写字母个数:" << upper << endl;
cout << "小写字母个数:" << lower << endl;
cout << "数字个数:" << digit << endl;
cout << "空格个数:" << space << endl;
cout << "其他字符个数:" << other << endl;
}
```
首先,在主函数中使用 `getline(cin, str)` 输入一行文字,然后调用 `count()` 函数进行统计。在 `count()` 函数中,使用循环遍历每个字符,然后使用 `isupper()`、`islower()`、`isdigit()`、`isspace()` 函数判断字符类型,最后统计出各类字符的个数并输出即可。
需要注意的是,这里使用了 `string` 类型来存储输入的一行文字,并且使用了函数参数传递。在实际应用中,可能需要考虑输入的字符串长度超过80个字符的情况,以及一些其他的异常情况。
相关推荐
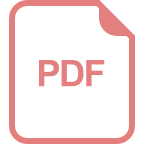
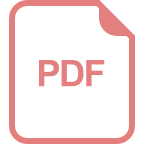
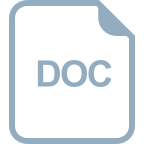
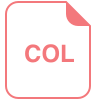
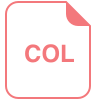
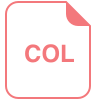
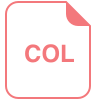
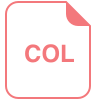





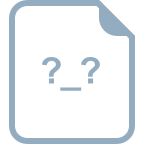
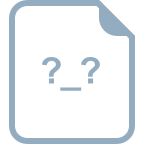
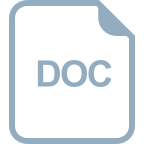