c++线程堆栈
时间: 2023-08-04 14:23:13 浏览: 300
在 C++11 标准中,C++ 提供了一个 std::thread 类来支持多线程编程。在创建一个 std::thread 对象时,可以通过构造函数传递一个可调用对象(如函数指针、函数对象等)和其参数,从而创建一个新的线程。
std::thread 对象的构造函数还可以接受一个可选的参数,用于指定线程堆栈的大小。例如,可以使用以下代码创建一个具有 2MB 堆栈大小的新线程:
```cpp
#include <iostream>
#include <thread>
void thread_func()
{
// 线程函数
}
int main()
{
std::thread my_thread(thread_func);
my_thread.join();
std::thread big_stack_thread(thread_func, 1024 * 1024 * 2);
big_stack_thread.join();
return 0;
}
```
在上面的代码中,第一个 std::thread 对象 my_thread 创建时没有指定堆栈大小,将使用默认值。第二个 std::thread 对象 big_stack_thread 创建时指定了堆栈大小为 2MB。
需要注意的是,线程堆栈的大小可能会影响程序的性能和稳定性。如果设置过小,可能会导致堆栈溢出错误;如果设置过大,可能会浪费系统资源。因此,在设置线程堆栈大小时需要谨慎,并根据实际情况进行调整。
相关问题
c++如何设置线程堆栈大小
在 C++ 中,可以使用线程属性来设置线程堆栈大小。具体步骤如下:
1. 定义线程属性对象: `pthread_attr_t attr;`
2. 初始化线程属性对象: `pthread_attr_init(&attr);`
3. 设置线程堆栈大小: `pthread_attr_setstacksize(&attr, stack_size);`,其中 `stack_size` 是堆栈大小,单位为字节。
4. 创建线程时,将线程属性对象传递给 `pthread_create` 函数: `pthread_create(&thread, &attr, start_routine, arg);`
5. 在不需要使用线程属性对象时,需要销毁线程属性对象: `pthread_attr_destroy(&attr);`
以下是一个简单的示例代码:
```c++
#include <pthread.h>
// 线程执行的函数
void* my_thread_func(void* arg) {
// TODO: 线程执行的逻辑
}
int main() {
pthread_t thread;
pthread_attr_t attr;
size_t stack_size = 1024 * 1024; // 堆栈大小为 1MB
// 初始化线程属性对象
pthread_attr_init(&attr);
// 设置线程堆栈大小
pthread_attr_setstacksize(&attr, stack_size);
// 创建线程,并传递线程属性对象
pthread_create(&thread, &attr, my_thread_func, NULL);
// 等待线程执行完成
pthread_join(thread, NULL);
// 销毁线程属性对象
pthread_attr_destroy(&attr);
return 0;
}
```
c++中std::thread如何设置线程堆栈大小
在 C++11 中,可以使用 `std::thread` 类来创建线程,并使用线程属性来设置线程堆栈大小。具体步骤如下:
1. 定义线程函数: `void my_thread_func();`
2. 创建线程属性对象: `std::thread::attributes_type attr;`
3. 设置线程堆栈大小: `attr.set_stack_size(stack_size);`,其中 `stack_size` 是堆栈大小,单位为字节。
4. 创建线程时,将线程属性对象传递给 `std::thread` 构造函数: `std::thread my_thread(my_thread_func, arg1, arg2, ..., std::move(attr));`
5. 在不需要使用线程属性对象时,线程析构函数会自动销毁线程属性对象。
以下是一个简单的示例代码:
```c++
#include <thread>
// 线程执行的函数
void my_thread_func() {
// TODO: 线程执行的逻辑
}
int main() {
std::thread::attributes_type attr;
size_t stack_size = 1024 * 1024; // 堆栈大小为 1MB
// 设置线程堆栈大小
attr.set_stack_size(stack_size);
// 创建线程,并传递线程属性对象
std::thread my_thread(my_thread_func, arg1, arg2, ..., std::move(attr));
// 等待线程执行完成
my_thread.join();
return 0;
}
```
需要注意的是,不同的操作系统和编译器可能对线程属性的支持不同,因此在使用时需要查阅相关文档进行确认。
阅读全文
相关推荐
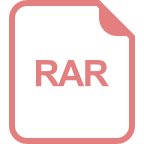
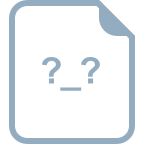
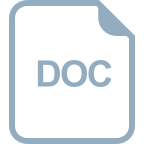
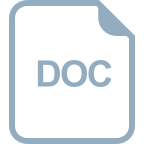
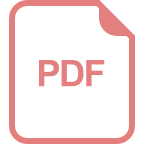
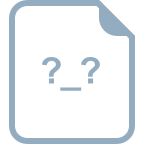
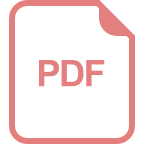
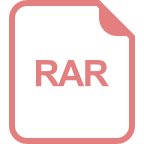
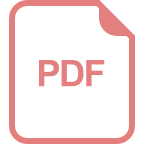
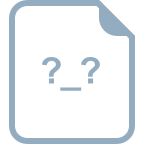
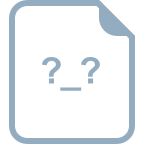
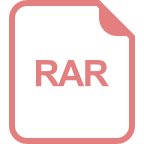
