C语言实现调用cgi.h,post实现开发实现登录验证
时间: 2024-04-22 10:28:46 浏览: 67
要实现 C 语言调用 CGI 接口,并通过 POST 方法实现登录验证,可以按照以下步骤进行:
1. 使用 C 语言编写客户端代码,调用 cURL 库进行网络请求。
2. 在客户端代码中,设置请求的 URL,POST 方法,请求头信息等参数。
3. 在请求体中,设置需要提交的表单数据,包括用户名和密码等信息。
4. 发送请求,并等待服务器返回响应。
5. 解析服务器返回的响应,判断登录是否成功。
下面是一个简单的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <curl/curl.h>
int main(void)
{
CURL *curl;
CURLcode res;
struct curl_slist *headerlist = NULL;
char *postdata = "username=test&password=123456";
char *url = "http://localhost/cgi-bin/login.cgi";
curl_global_init(CURL_GLOBAL_ALL);
curl = curl_easy_init();
if (curl) {
headerlist = curl_slist_append(headerlist, "Content-Type: application/x-www-form-urlencoded");
curl_easy_setopt(curl, CURLOPT_URL, url);
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, postdata);
curl_easy_setopt(curl, CURLOPT_POSTFIELDSIZE, strlen(postdata));
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headerlist);
res = curl_easy_perform(curl);
if (res != CURLE_OK) {
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res));
}
curl_easy_cleanup(curl);
curl_slist_free_all(headerlist);
}
curl_global_cleanup();
return 0;
}
```
在上述代码中,我们使用了 cURL 库来发送 HTTP 请求,并设置了请求的 URL、POST 方法、请求体等参数。同时,我们还定义了一个请求头信息的链表和一个请求体字符串。需要注意的是,上述代码中的“http://localhost/cgi-bin/login.cgi”应改为实际的 CGI 脚本的 URL。同时,需要根据实际情况修改表单数据中的用户名和密码等信息。
阅读全文
相关推荐
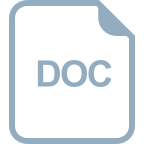
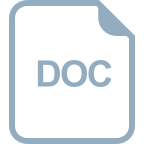
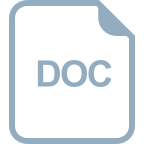

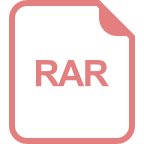

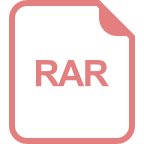
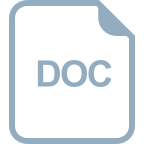
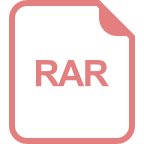
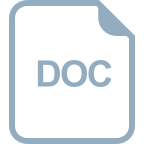
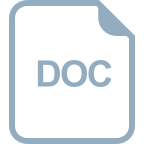
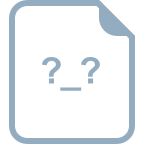
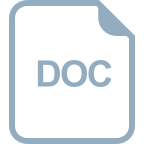
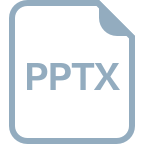
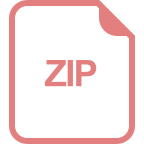
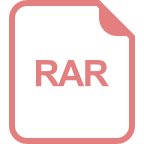
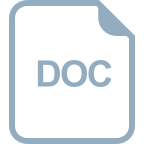
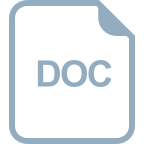
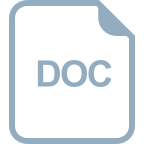
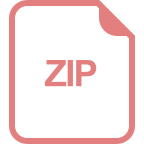