python如何画9*9数独表
时间: 2023-11-16 12:32:33 浏览: 29
你可以使用 Python 的第三方库 `pygame` 来画出 9*9 数独表。以下是一个示例代码:
```python
import pygame
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GRAY = (128, 128, 128)
# 初始化 Pygame
pygame.init()
# 设置窗口大小和标题
size = (540, 600)
screen = pygame.display.set_mode(size)
pygame.display.set_caption("数独表")
# 设置字体
font = pygame.font.SysFont('fangsong', 40)
# 定义要填入的数独表
sudoku = [
[0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0]
]
# 定义数独表的单元格位置和大小
cell_width = 60
cell_height = 60
cell_margin = 5
# 定义数独表的位置
x_offset = 30
y_offset = 60
# 游戏循环
done = False
while not done:
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
# 清屏
screen.fill(WHITE)
# 画数独表格子
for row in range(9):
for col in range(9):
# 计算单元格位置
x = x_offset + col * (cell_width + cell_margin)
y = y_offset + row * (cell_height + cell_margin)
# 画单元格
pygame.draw.rect(screen, GRAY, [x, y, cell_width, cell_height])
# 如果单元格有值,就在单元格中央显示该值
value = str(sudoku[row][col])
if value != "0":
text = font.render(value, True, BLACK)
text_rect = text.get_rect(center=(x + cell_width / 2, y + cell_height / 2))
screen.blit(text, text_rect)
# 更新屏幕
pygame.display.flip()
# 退出 Pygame
pygame.quit()
```
运行上述代码,即可显示一个空的 9*9 数独表。你可以在代码中修改 `sudoku` 变量的值来显示不同的数独题目。
相关推荐
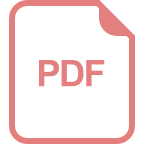














