nestjs 实现post请求第三方接口
时间: 2024-05-25 09:04:53 浏览: 19
要使用 NestJS 实现 POST 请求第三方接口,可以按照以下步骤进行:
1. 首先需要安装 `axios` 模块,它是一个基于 Promise 的 HTTP 客户端,用于浏览器和 Node.js。
```bash
npm i axios
```
2. 在需要调用第三方接口的地方,引入 `axios` 模块。
```typescript
import axios from 'axios';
```
3. 使用 `axios` 模块发起 POST 请求。
```typescript
async function postToThirdParty(url: string, data: any): Promise<any> {
try {
const response = await axios.post(url, data);
return response.data;
} catch (error) {
console.error(error);
return null;
}
}
```
在上面的代码中,`postToThirdParty` 函数接收两个参数:第一个是第三方接口 URL,第二个是 POST 请求中的数据。函数通过调用 `axios.post` 方法发起 POST 请求,并返回响应数据。如果请求失败,则函数返回 `null`。
4. 在 NestJS 控制器中调用 `postToThirdParty` 函数。
```typescript
import { Controller, Post, Body } from '@nestjs/common';
@Controller('third-party')
export class ThirdPartyController {
@Post()
async postToThirdParty(@Body() data: any): Promise<any> {
const url = 'https://third.party.api';
const response = await postToThirdParty(url, data);
return response;
}
}
```
在上面的代码中,我们创建了一个名为 `ThirdPartyController` 的 NestJS 控制器,并添加了一个 `postToThirdParty` 方法。该方法使用 `@Post` 装饰器将其定义为 POST 请求处理程序。`@Body` 装饰器用于将请求体中的数据作为参数传递给该方法。在方法体中,我们调用了 `postToThirdParty` 函数,并返回响应数据。
这样就可以使用 NestJS 实现 POST 请求第三方接口了。
相关推荐
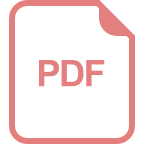













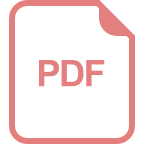
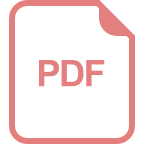