Java代码实现Trie字典树查询商品
时间: 2023-06-03 11:03:28 浏览: 88
下面是一个Java代码示例,实现了Trie字典树来查询商品:
```
import java.util.HashMap;
import java.util.Map;
class TrieNode {
Map<Character, TrieNode> children;
boolean isEndOfWord;
String item;
public TrieNode() {
children = new HashMap<>();
isEndOfWord = false;
}
}
class Trie {
TrieNode root;
public Trie() {
root = new TrieNode();
}
public void insert(String item, String name) {
TrieNode current = root;
for (int i = 0; i < item.length(); i++) {
char ch = item.charAt(i);
TrieNode node = current.children.get(ch);
if (node == null) {
node = new TrieNode();
current.children.put(ch, node);
}
current = node;
}
current.isEndOfWord = true;
current.item = name;
}
public String search(String item) {
TrieNode current = root;
for (int i = 0; i < item.length(); i++) {
char ch = item.charAt(i);
TrieNode node = current.children.get(ch);
if (node == null) {
return null;
}
current = node;
}
if (current.isEndOfWord) {
return current.item;
} else {
return null;
}
}
}
```
上面的代码实现了一个Trie字典树类,其中包含了插入商品和查询商品的功能。
在插入商品的时候,把商品名称插入到字典树中,在查询商品的时候,查询商品名称是否存在于字典树中。
这样通过Trie字典树来实现商品查询是一个很高效的方法,在查询时间复杂度为O(n),n为商品名称的长度.
相关推荐
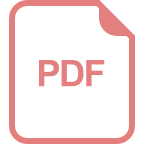















