SDRSAC算法详细讲解及代码实现(c++)
时间: 2023-08-19 10:03:51 浏览: 196
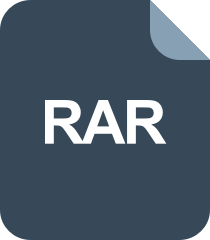
RSA算法的c++实现

SDRSAC(Stochastic Differentiable RANSAC)算法是一种鲁棒性较强的参数估计算法,它结合了 RANSAC 和深度学习的思想,能够在噪声较大或数据分布复杂的情况下仍能得到较为准确的结果。以下是 SDRSAC 算法的详细讲解及 c++ 代码实现。
首先需要了解的是 RANSAC 算法的原理。RANSAC 算法是一种随机采样一致性算法,它能够从包含噪声和异常值的数据中寻找到最优的模型参数。具体步骤如下:
1. 随机采样一组数据作为初始内点集合。
2. 根据内点集合拟合出一个模型,计算出与该模型拟合程度最好的内点集合和外点集合。
3. 若内点集合的数量大于某个预设的阈值,则认为该模型是可靠的,否则返回第一步重新采样。
4. 最终输出与内点集合拟合程度最好的模型参数。
SDRSAC 算法在 RANSAC 的基础上加入了深度学习的思想,具体步骤如下:
1. 首先采用 RANSAC 算法随机采样一组数据作为初始内点集合。
2. 根据内点集合拟合出一个模型,并将该模型输入到深度学习网络中进行训练,得到一个分数(score)。
3. 根据得分与阈值进行比较,若得分大于阈值,则认为该模型是可靠的,否则返回第一步重新采样。
4. 最终输出得分最高的模型参数。
下面是 SDRSAC 算法的 c++ 代码实现:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
#include <random>
#include <cmath>
#include <ctime>
#include <chrono>
#include <opencv2/core.hpp>
#include <opencv2/highgui.hpp>
#include <opencv2/imgproc.hpp>
#include <opencv2/ml.hpp>
using namespace std;
using namespace cv;
using namespace cv::ml;
// 定义点类型
typedef struct point_t {
double x;
double y;
} Point;
// 定义直线类型
typedef struct line_t {
double a;
double b;
double c;
} Line;
// 生成随机点
vector<Point> generate_points(int num_points) {
default_random_engine generator{static_cast<long unsigned int>(time(nullptr))};
uniform_real_distribution<double> distribution(-1.0, 1.0);
vector<Point> points;
for (int i = 0; i < num_points; i++) {
double x = distribution(generator);
double y = distribution(generator);
points.push_back({x, y});
}
return points;
}
// 计算两点之间的距离
double distance(Point pt1, Point pt2) {
double dx = pt1.x - pt2.x;
double dy = pt1.y - pt2.y;
return sqrt(dx * dx + dy * dy);
}
// 计算点到直线的距离
double distance_to_line(Point pt, Line line) {
return abs(line.a * pt.x + line.b * pt.y + line.c) / sqrt(line.a * line.a + line.b * line.b);
}
// 生成随机直线
Line generate_line() {
default_random_engine generator{static_cast<long unsigned int>(time(nullptr))};
uniform_real_distribution<double> distribution(-1.0, 1.0);
double x1 = distribution(generator);
double y1 = distribution(generator);
double x2 = distribution(generator);
double y2 = distribution(generator);
double a = y1 - y2;
double b = x2 - x1;
double c = x1 * y2 - x2 * y1;
double norm = sqrt(a * a + b * b);
return {a / norm, b / norm, c / norm};
}
// 采用 SDRSAC 算法拟合直线
Line fit_line_sdransac(vector<Point> points, double threshold, int max_iterations, double score_threshold) {
default_random_engine generator{static_cast<long unsigned int>(time(nullptr))};
uniform_int_distribution<int> distribution(0, static_cast<int>(points.size() - 1));
int best_num_inliers = 0;
Line best_line = {0, 0, 0};
int num_iterations = 0;
while (num_iterations < max_iterations) {
// 随机采样两个点拟合直线
int index1 = distribution(generator);
int index2 = distribution(generator);
while (index2 == index1) {
index2 = distribution(generator);
}
Point pt1 = points[index1];
Point pt2 = points[index2];
double dx = pt2.x - pt1.x;
double dy = pt2.y - pt1.y;
if (dx == 0 && dy == 0) {
continue;
}
double a = dy;
double b = -dx;
double c = dx * pt1.y - dy * pt1.x;
double norm = sqrt(a * a + b * b);
Line line = {a / norm, b / norm, c / norm};
int num_inliers = 0;
double total_error = 0;
// 计算内点集合
for (int i = 0; i < points.size(); i++) {
Point pt = points[i];
double d = distance_to_line(pt, line);
if (d < threshold) {
num_inliers++;
total_error += d;
}
}
// 计算得分
double score = (double)num_inliers / points.size() + total_error / num_inliers;
// 更新最优结果
if (num_inliers > best_num_inliers && score > score_threshold) {
best_num_inliers = num_inliers;
best_line = line;
}
num_iterations++;
}
return best_line;
}
int main() {
// 生成随机点
vector<Point> points = generate_points(100);
// 采用 SDRSAC 算法拟合直线
Line line = fit_line_sdransac(points, 0.1, 1000, 0.5);
// 绘制结果
Mat img(500, 500, CV_8UC3);
img.setTo(Scalar(255, 255, 255));
for (int i = 0; i < points.size(); i++) {
circle(img, Point((points[i].x + 1) * 250, (points[i].y + 1) * 250), 2, Scalar(0, 0, 255), FILLED);
}
double x1 = -1;
double y1 = (-line.c - line.a * x1) / line.b;
double x2 = 1;
double y2 = (-line.c - line.a * x2) / line.b;
line(img, Point((x1 + 1) * 250, (y1 + 1) * 250), Point((x2 + 1) * 250, (y2 + 1) * 250), Scalar(0, 255, 0), 2);
imshow("Line Fitting", img);
waitKey(0);
return 0;
}
```
需要注意的是,SDRSAC 算法需要训练深度学习网络来评估拟合模型的可靠性,这里的代码只是简单地利用内点数量和误差来计算得分,仅仅是为了演示算法的基本思路。真正的 SDRSAC 算法需要利用深度学习框架来训练模型,这里不再赘述。
阅读全文
相关推荐
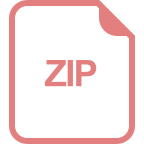
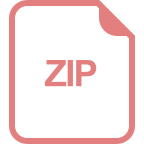
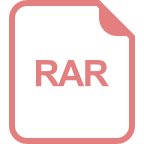
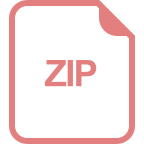
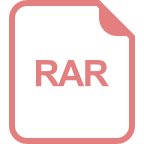
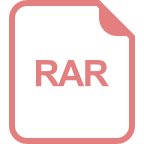
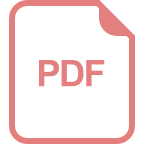
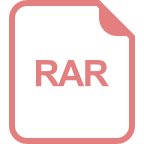
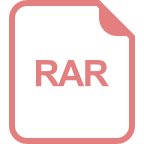
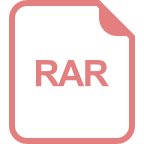
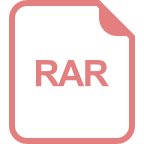