用vue写图书管理系统
时间: 2023-11-30 21:32:19 浏览: 36
好的,下面是一个简单的图书管理系统的 Vue 实现。在这个系统中,我们可以添加、编辑、删除和搜索图书。
首先,我们需要创建一个 Vue 实例,并在实例中定义需要的数据和方法。
```html
<template>
<div>
<h1>图书管理系统</h1>
<div>
<input type="text" v-model="search" placeholder="搜索书名或作者" />
<button @click="searchBooks">搜索</button>
</div>
<div>
<h2>图书列表</h2>
<table>
<thead>
<tr>
<th>书名</th>
<th>作者</th>
<th>出版社</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(book, index) in books" :key="book.id">
<td>{{ book.name }}</td>
<td>{{ book.author }}</td>
<td>{{ book.publisher }}</td>
<td>
<button @click="editBook(index)">编辑</button>
<button @click="deleteBook(index)">删除</button>
</td>
</tr>
</tbody>
</table>
<button @click="addBook">添加图书</button>
</div>
<div v-if="showAddBook">
<h2>添加图书</h2>
<form @submit.prevent="addNewBook">
<div>
<label>书名:</label>
<input type="text" v-model="newBook.name" />
</div>
<div>
<label>作者:</label>
<input type="text" v-model="newBook.author" />
</div>
<div>
<label>出版社:</label>
<input type="text" v-model="newBook.publisher" />
</div>
<button type="submit">添加</button>
<button @click="cancelAddBook">取消</button>
</form>
</div>
<div v-if="showEditBook">
<h2>编辑图书</h2>
<form @submit.prevent="updateBook">
<div>
<label>书名:</label>
<input type="text" v-model="selectedBook.name" />
</div>
<div>
<label>作者:</label>
<input type="text" v-model="selectedBook.author" />
</div>
<div>
<label>出版社:</label>
<input type="text" v-model="selectedBook.publisher" />
</div>
<button type="submit">保存</button>
<button @click="cancelEditBook">取消</button>
</form>
</div>
</div>
</template>
<script>
export default {
data() {
return {
books: [
{
id: 1,
name: "Vue.js 实战",
author: "梁灏",
publisher: "人民邮电出版社"
},
{
id: 2,
name: "JavaScript高级程序设计",
author: "Nicholas C. Zakas",
publisher: "人民邮电出版社"
},
{
id: 3,
name: "深入浅出Node.js",
author: "朴灵",
publisher: "人民邮电出版社"
}
],
search: "",
showAddBook: false,
showEditBook: false,
newBook: {
name: "",
author: "",
publisher: ""
},
selectedBook: {}
};
},
methods: {
searchBooks() {
// 搜索图书
// 根据书名或作者进行模糊匹配
if (this.search.trim() === "") {
return;
}
this.books = this.books.filter(
book =>
book.name.includes(this.search) || book.author.includes(this.search)
);
},
addBook() {
// 显示添加图书表单
this.showAddBook = true;
},
addNewBook() {
// 添加新图书
const maxId = Math.max(...this.books.map(book => book.id));
this.newBook.id = maxId + 1;
this.books.push(this.newBook);
this.newBook = {
name: "",
author: "",
publisher: ""
};
this.showAddBook = false;
},
cancelAddBook() {
// 取消添加图书
this.showAddBook = false;
this.newBook = {
name: "",
author: "",
publisher: ""
};
},
editBook(index) {
// 显示编辑图书表单
this.selectedBook = { ...this.books[index] };
this.showEditBook = true;
},
updateBook() {
// 更新图书信息
const index = this.books.findIndex(
book => book.id === this.selectedBook.id
);
this.books.splice(index, 1, this.selectedBook);
this.selectedBook = {};
this.showEditBook = false;
},
cancelEditBook() {
// 取消编辑图书
this.selectedBook = {};
this.showEditBook = false;
},
deleteBook(index) {
// 删除图书
if (confirm("确定删除该图书?")) {
this.books.splice(index, 1);
}
}
}
};
</script>
```
在这个例子中,我们使用了 `v-for` 指令来遍历图书列表,使用 `v-model` 指令来实现表单数据双向绑定,使用 `@click` 指令来监听按钮点击事件,使用 `v-if` 指令来控制表单的显示和隐藏。同时,我们还定义了一些方法来实现添加、编辑、删除和搜索图书的功能。
完整代码可以在 CodePen 上查看:https://codepen.io/pen/?template=JjWwOwK
相关推荐
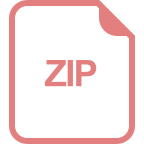
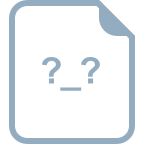










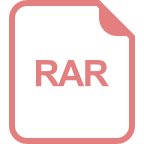
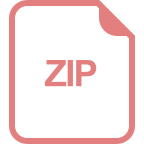
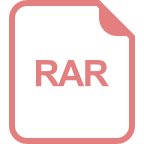