@staticmethod def inference_detection(image, train=False): """ HandSegNet: Detects the hand in the input image by segmenting it. Inputs: image: [B, H, W, 3] tf.float32 tensor, Image with mean subtracted train: bool, True in case weights should be trainable Outputs: scoremap_list_large: list of [B, 256, 256, 2] tf.float32 tensor, Scores for the hand segmentation classes """ with tf.compat.v1.variable_scope('HandSegNet'): scoremap_list = list() layers_per_block = [2, 2, 4, 4] out_chan_list = [64, 128, 256, 512] pool_list = [True, True, True, False] # learn some feature representation, that describes the image content well x = image for block_id, (layer_num, chan_num, pool) in enumerate(zip(layers_per_block, out_chan_list, pool_list), 1): for layer_id in range(layer_num): x = ops.conv_relu(x, 'conv%d_%d' % (block_id, layer_id+1), kernel_size=3, stride=1, out_chan=chan_num, trainable=train) if pool: x = ops.max_pool(x, 'pool%d' % block_id) x = ops.conv_relu(x, 'conv5_1', kernel_size=3, stride=1, out_chan=512, trainable=train) encoding = ops.conv_relu(x, 'conv5_2', kernel_size=3, stride=1, out_chan=128, trainable=train) # use encoding to detect initial scoremap x = ops.conv_relu(encoding, 'conv6_1', kernel_size=1, stride=1, out_chan=512, trainable=train) scoremap = ops.conv(x, 'conv6_2', kernel_size=1, stride=1, out_chan=2, trainable=train) scoremap_list.append(scoremap) # upsample to full size s = image.get_shape().as_list() scoremap_list_large = [tf.image.resize_images(x, (s[1], s[2])) for x in scoremap_list] return scoremap_list_large详细注释
时间: 2023-12-06 16:02:46 浏览: 32
这段代码是一个静态方法,用于对输入的图像进行手部分割,返回一个列表,包含了手部分割的分数图。其中,输入参数包括一个形状为[B, H, W, 3]的tf.float32类型的图像张量,train参数用于指定权重是否可训练。输出为一个形状为[B, 256, 256, 2]的tf.float32类型的列表,其中每个元素是手部分割的分数图。这段代码的实现主要是通过卷积和池化操作来学习图像的特征表示,并利用学到的特征表示来进行手部分割。具体实现包括了5个卷积层和1个上采样层,其中前4个卷积层包含了不同的卷积核数目和卷积层数,最后一个卷积层和上采样层用于生成手部分割的分数图。
相关问题
class UserSigninLog(db.Model): """登录日志表""" bind_key = 'db2' tablename = 'user_signin_log' id = mapped_column(Integer, primary_key=True) userid = mapped_column(String(16), index=True) username = mapped_column(String(32)) ip_addr = mapped_column(String(32)) request_user_agent = mapped_column(String(256)) c_date = mapped_column(DateTime) @staticmethod def add_user_signin_log(userid: str, username: str): """新增登录日志""" user_signin_log = UserSigninLog() user_signin_log.userid = userid user_signin_log.username = username user_signin_log.ip_addr = request.access_route[0] user_signin_log.request_user_agent = request.headers.get("User-Agent") user_signin_log.c_date = datetime.now() db.session.add(user_signin_log) db.session.commit() return True @staticmethod def check_ip_and_header_within_x_days(userid: str, days=7): """检查x天内登录过的ip地址和请求头""" sql_query = select(UserSigninLog) \ .where(UserSigninLog.userid == userid, UserSigninLog.ip_addr == request.access_route[0], UserSigninLog.request_user_agent == request.headers.get("User-Agent"), UserSigninLog.c_date >= datetime.now() - timedelta(days=days)).limit(1) user_ip_addr_log = db.session.execute(sql_query).scalar_one_or_none() if user_ip_addr_log: return True return False 请帮我建议并优化这段代码,python3.8
以下是我对这段代码的建议和优化:
1. 使用 Flask 的上下文对象获取 request 对象,避免在静态方法中使用全局变量
```python
from flask import request
class UserSigninLog(db.Model):
# ...
@staticmethod
def add_user_signin_log(userid: str, username: str):
"""新增登录日志"""
user_signin_log = UserSigninLog()
user_signin_log.userid = userid
user_signin_log.username = username
user_signin_log.ip_addr = request.access_route[0]
user_signin_log.request_user_agent = request.headers.get("User-Agent")
user_signin_log.c_date = datetime.now()
db.session.add(user_signin_log)
db.session.commit()
return True
@staticmethod
def check_ip_and_header_within_x_days(userid: str, days=7):
"""检查x天内登录过的ip地址和请求头"""
user_agent = request.headers.get("User-Agent")
ip_addr = request.access_route[0]
c_date = datetime.now() - timedelta(days=days)
user_ip_addr_log = UserSigninLog.query.filter_by(userid=userid, ip_addr=ip_addr, request_user_agent=user_agent).filter(UserSigninLog.c_date >= c_date).first()
if user_ip_addr_log:
return True
return False
```
2. 使用 SQLAlchemy 的 ORM 语法查询,避免手写 SQL 语句
```python
from sqlalchemy import and_
class UserSigninLog(db.Model):
# ...
@staticmethod
def check_ip_and_header_within_x_days(userid: str, days=7):
"""检查x天内登录过的ip地址和请求头"""
user_agent = request.headers.get("User-Agent")
ip_addr = request.access_route[0]
c_date = datetime.now() - timedelta(days=days)
user_ip_addr_log = UserSigninLog.query.filter(and_(UserSigninLog.userid == userid, UserSigninLog.ip_addr == ip_addr, UserSigninLog.request_user_agent == user_agent, UserSigninLog.c_date >= c_date)).first()
if user_ip_addr_log:
return True
return False
```
3. 让静态方法更加灵活,接收 request 对象作为参数
```python
class UserSigninLog(db.Model):
# ...
@staticmethod
def add_user_signin_log(userid: str, username: str, request):
"""新增登录日志"""
user_signin_log = UserSigninLog()
user_signin_log.userid = userid
user_signin_log.username = username
user_signin_log.ip_addr = request.access_route[0]
user_signin_log.request_user_agent = request.headers.get("User-Agent")
user_signin_log.c_date = datetime.now()
db.session.add(user_signin_log)
db.session.commit()
return True
@staticmethod
def check_ip_and_header_within_x_days(userid: str, request, days=7):
"""检查x天内登录过的ip地址和请求头"""
user_agent = request.headers.get("User-Agent")
ip_addr = request.access_route[0]
c_date = datetime.now() - timedelta(days=days)
user_ip_addr_log = UserSigninLog.query.filter(and_(UserSigninLog.userid == userid, UserSigninLog.ip_addr == ip_addr, UserSigninLog.request_user_agent == user_agent, UserSigninLog.c_date >= c_date)).first()
if user_ip_addr_log:
return True
return False
```
这些是我对这段代码的建议和优化,希望能对你有所帮助。
@staticmethod def inter_set(a: set, b: set) -> bool: if a.intersection(b) and a.intersection(b) <= a: return True else: return False修改为python2.7可以识别的
在Python 2.7中,类型注解是无法被正确识别的,因此需要将其去掉。另外,Python 2.7中的print语句需要加上括号。修改后的代码如下:
```python
class SetUtils(object):
@staticmethod
def inter_set(a, b):
if a.intersection(b) and a.intersection(b) <= a:
return True
else:
return False
```
在Python 2.7中,需要在类定义中显式地继承object。同时,由于Python 2.7中的set类型并不是内置类型,而是需要从sets模块中导入,因此在使用set时需要先导入sets模块。
相关推荐
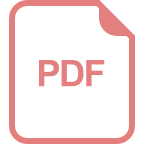
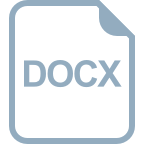
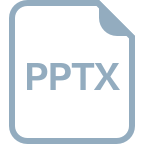












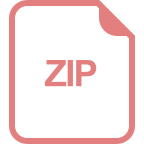