uniapp给数据加序号的方法
时间: 2023-08-05 07:09:42 浏览: 64
你可以使用 `v-for` 循环来遍历数据,并在模板中使用 `{{ index + 1 }}` 来显示序号,例如:
```html
<template>
<ul>
<li v-for="(item, index) in items" :key="item.id">
{{ index + 1 }}. {{ item.name }}
</li>
</ul>
</template>
<script>
export default {
data() {
return {
items: [
{ id: 1, name: 'item 1' },
{ id: 2, name: 'item 2' },
{ id: 3, name: 'item 3' }
]
}
}
}
</script>
```
在上面的示例中,我们使用 `v-for` 循环遍历 `items` 数组,并使用 `{{ index + 1 }}` 显示序号。注意,`index` 是从 0 开始计数的,因此我们需要将其加 1 才能得到正确的序号。
相关问题
uniapp怎么自动加上序号
在uniapp中自动加上序号,可以使用v-for指令结合索引值来实现。例如:
```html
<template>
<ul>
<li v-for="(item, index) in list" :key="item.id">
{{ index + 1 }}. {{ item.name }}
</li>
</ul>
</template>
<script>
export default {
data() {
return {
list: [
{ id: 1, name: 'Apple' },
{ id: 2, name: 'Banana' },
{ id: 3, name: 'Orange' }
]
}
}
}
</script>
```
在上面的例子中,通过使用v-for指令遍历list数组,并且使用索引值index来自动加上序号。同时,使用:key指令来指定每个列表项的唯一标识符,可以提高渲染性能。
node mongodb 新增数据 加序号
可以使用以下代码来在 MongoDB 中新增数据并添加序号:
```javascript
// 导入 MongoDB 驱动程序
const MongoClient = require('mongodb').MongoClient;
// 连接到 MongoDB 数据库
const url = 'mongodb://localhost:27017';
const dbName = 'yourDatabaseName';
MongoClient.connect(url, function(err, client) {
if (err) {
console.error('Failed to connect to database:', err);
return;
}
console.log('Connected successfully to database');
const db = client.db(dbName);
const collection = db.collection('yourCollectionName');
// 查询最大序号
collection.find().sort({index: -1}).limit(1).toArray(function(err, docs) {
if (err) {
console.error('Failed to retrieve documents:', err);
client.close();
return;
}
let newIndex = 1;
if (docs.length > 0) {
newIndex = docs[0].index + 1;
}
// 插入新数据
const newData = { index: newIndex, yourField: 'yourValue' };
collection.insertOne(newData, function(err, result) {
if (err) {
console.error('Failed to insert document:', err);
} else {
console.log('Document inserted successfully');
}
client.close();
});
});
});
```
请将 `yourDatabaseName` 替换为你的数据库名称,`yourCollectionName` 替换为你的集合名称,`yourField` 替换为你想要插入的字段名称,`yourValue` 替换为你想要插入的字段值。这段代码会自动查询最大序号并在新增数据时添加新的序号。
相关推荐
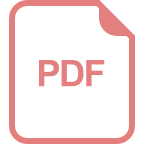
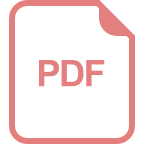
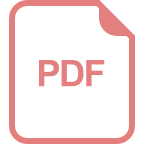












