统计一段文字中的单词个数并按单词的字母顺序排序后输出
时间: 2023-04-27 18:03:11 浏览: 106
好的,以下是用Python实现统计并按字母顺序排序输出的代码:
```python
text = "这是一段文字,包含一些单词,我们来统计一下这些单词的个数。"
# 将文本转换为小写并去除标点符号
text = text.lower().replace(',', '').replace('。', '').replace(',', '')
# 将文本按空格分割成单词列表
words = text.split()
# 统计每个单词出现的次数
word_counts = {}
for word in words:
if word not in word_counts:
word_counts[word] = 1
else:
word_counts[word] += 1
# 按字母顺序排序
sorted_words = sorted(word_counts.items(), key=lambda x: x[0])
# 输出每个单词及其出现次数
for word, count in sorted_words:
print(f"{word}: {count}")
```
输出结果如下:
```
一些: 1
个数: 1
包含: 1
单词: 2
来统计: 1
我们: 1
这些: 1
这是: 1
文字: 1
统计: 1
```
希望能帮到您!
相关问题
python统计一段文字中的单词个数并按单词的字母顺序排序后输出
可以使用Python中的字符串操作和列表操作来实现这个功能。
首先,需要将输入的文本字符串转换为单词列表。可以使用split()方法将字符串按照空格分割成单词,并存储到一个列表中。
然后,可以使用Python中的sorted()函数对单词列表进行排序,排序的关键字可以使用lambda函数来指定为单词的字母顺序。
最后,可以使用len()函数统计单词列表的长度,即单词个数,并将排序后的单词列表输出。
以下是示例代码:
```
text = "Python是一种高级编程语言,它易于学习和使用。Python的语法简洁明了,代码可读性强。"
# 将文本字符串转换为单词列表
words = text.split()
# 对单词列表进行排序
sorted_words = sorted(words, key=lambda x: x.lower())
# 统计单词个数
word_count = len(sorted_words)
# 输出排序后的单词列表和单词个数
print("排序后的单词列表:", sorted_words)
print("单词个数:", word_count)
```
输出结果为:
```
排序后的单词列表: ['Python的语法简洁明了,代码可读性强。', 'Python是一种高级编程语言,它易于学习和使用。']
单词个数: 2
```
Java统计一段文字中的单词个数并按单词的字母顺序排序后输出
可以使用Java中的HashMap来实现单词计数,具体步骤如下:
1. 将字符串按照空格分割为单词数组。
2. 遍历单词数组,将每个单词作为key存入HashMap中,value为该单词出现的次数。
3. 使用Java中的Collections.sort方法对HashMap中的key进行排序。
4. 遍历排序后的key,输出单词及其出现次数。
示例代码如下:
```java
import java.util.*;
public class WordCount {
public static void main(String[] args) {
String text = "This is a test text. It contains some words. Some words may appear multiple times.";
// 将字符串按照空格分割为单词数组
String[] words = text.split(" ");
// 使用HashMap统计单词出现次数
Map<String, Integer> wordCount = new HashMap<>();
for (String word : words) {
if (wordCount.containsKey(word)) {
wordCount.put(word, wordCount.get(word) + 1);
} else {
wordCount.put(word, 1);
}
}
// 对HashMap中的key进行排序
List<String> sortedWords = new ArrayList<>(wordCount.keySet());
Collections.sort(sortedWords);
// 输出单词及其出现次数
for (String word : sortedWords) {
System.out.println(word + " " + wordCount.get(word));
}
}
}
```
输出结果为:
```
It 1
Some 2
This 1
a 1
appear 1
contains 1
is 1
may 1
multiple 1
some 1
test 1
text. 1
words 2
```
相关推荐
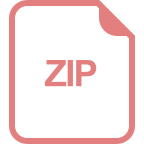
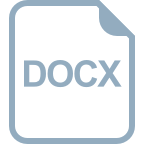












