利用stm32F401VD的PC0-PC15写一个8×4的矩阵键盘代码
时间: 2024-05-03 22:21:30 浏览: 92
以下是基于STM32F401VD的PC0-PC15的8x4矩阵键盘代码:
首先,需要在STM32CubeMX中配置GPIO端口为输入模式,并启用上拉电阻。接下来,可以使用HAL库和GPIO库来编写代码。
```c
#include "stm32f4xx_hal.h"
#include "gpio.h"
#define ROWS 4
#define COLS 8
GPIO_TypeDef* rowPorts[ROWS] = {GPIOC, GPIOC, GPIOC, GPIOC};
uint16_t rowPins[ROWS] = {GPIO_PIN_0, GPIO_PIN_1, GPIO_PIN_2, GPIO_PIN_3};
GPIO_TypeDef* colPorts[COLS] = {GPIOC, GPIOC, GPIOC, GPIOC, GPIOC, GPIOC, GPIOC, GPIOC};
uint16_t colPins[COLS] = {GPIO_PIN_4, GPIO_PIN_5, GPIO_PIN_6, GPIO_PIN_7, GPIO_PIN_8, GPIO_PIN_9, GPIO_PIN_10, GPIO_PIN_11};
// 检查按键是否按下
uint8_t isKeyPressed(uint8_t row, uint8_t col) {
HAL_GPIO_WritePin(rowPorts[row], rowPins[row], GPIO_PIN_RESET);
uint8_t state = HAL_GPIO_ReadPin(colPorts[col], colPins[col]);
HAL_GPIO_WritePin(rowPorts[row], rowPins[row], GPIO_PIN_SET);
return !state;
}
int main(void) {
HAL_Init();
SystemClock_Config();
MX_GPIO_Init();
// 设置所有行为输出,列为输入
for (int i = 0; i < ROWS; i++) {
HAL_GPIO_WritePin(rowPorts[i], rowPins[i], GPIO_PIN_SET);
GPIO_InitTypeDef GPIO_InitStruct = {0};
GPIO_InitStruct.Pin = rowPins[i];
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Pull = GPIO_PULLUP;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(rowPorts[i], &GPIO_InitStruct);
}
for (int i = 0; i < COLS; i++) {
GPIO_InitTypeDef GPIO_InitStruct = {0};
GPIO_InitStruct.Pin = colPins[i];
GPIO_InitStruct.Mode = GPIO_MODE_INPUT;
GPIO_InitStruct.Pull = GPIO_PULLUP;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(colPorts[i], &GPIO_InitStruct);
}
while (1) {
// 扫描矩阵
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (isKeyPressed(i, j)) {
// 处理按键事件
}
}
}
}
}
```
在主循环中,使用`isKeyPressed()`函数来检查按键是否按下。这个函数首先将行设置为低电平,然后读取列的状态。如果列为低电平,则说明按键被按下。
在初始化代码中,将所有行设置为输出模式,并将它们设置为高电平,将所有列设置为输入模式,并启用上拉电阻。这样,当行被设置为低电平时,将会通过列来检测按键状态。
注意:这个代码仅作为示例,需要根据实际硬件配置进行修改。
阅读全文
相关推荐
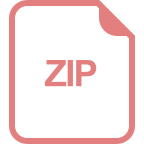
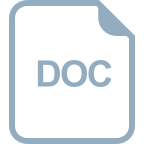


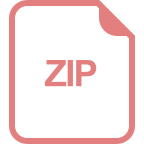
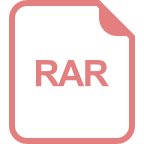
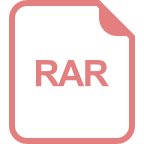
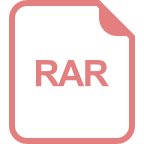
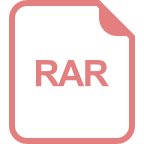
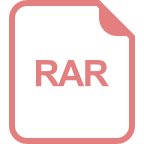
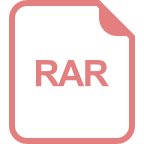
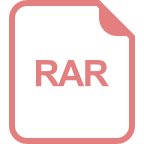
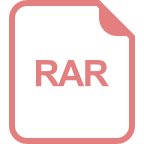
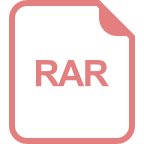
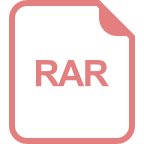
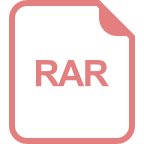