获取SQL server数据库中所有表的数据 C++实现
时间: 2024-03-21 09:41:04 浏览: 23
你可以使用 SQL Server 提供的系统存储过程 sp_MSforeachtable 来遍历所有表,并使用 SELECT 语句获取每个表的数据。以下是一个 C++ 实现的示例代码:
```cpp
#include <iostream>
#include <sql.h>
#include <sqlext.h>
#define SQL_RESULT_LEN 240
#define SQL_RETURN_CODE_LEN 1000
using namespace std;
int main() {
// Declare variables
SQLHANDLE sqlenvhandle;
SQLHANDLE sqlconnectionhandle;
SQLHANDLE sqlstatementhandle;
SQLRETURN retcode;
SQLCHAR retconstring[SQL_RETURN_CODE_LEN];
// Allocate environment handle
retcode = SQLAllocHandle(SQL_HANDLE_ENV, SQL_NULL_HANDLE, &sqlenvhandle);
// Set the ODBC version environment attribute
if (retcode == SQL_SUCCESS || retcode == SQL_SUCCESS_WITH_INFO) {
retcode = SQLSetEnvAttr(sqlenvhandle, SQL_ATTR_ODBC_VERSION, (SQLPOINTER)SQL_OV_ODBC3, 0);
// Allocate connection handle
if (retcode == SQL_SUCCESS || retcode == SQL_SUCCESS_WITH_INFO) {
retcode = SQLAllocHandle(SQL_HANDLE_DBC, sqlenvhandle, &sqlconnectionhandle);
// Set the connection timeout attribute
if (retcode == SQL_SUCCESS || retcode == SQL_SUCCESS_WITH_INFO) {
SQLSetConnectAttr(sqlconnectionhandle, SQL_LOGIN_TIMEOUT, (SQLPOINTER)5, 0);
// Connect to SQL Server
retcode = SQLConnect(sqlconnectionhandle, (SQLCHAR*)"your_server_name", SQL_NTS, (SQLCHAR*)"your_username", SQL_NTS, (SQLCHAR*)"your_password", SQL_NTS);
// Allocate statement handle
if (retcode == SQL_SUCCESS || retcode == SQL_SUCCESS_WITH_INFO) {
retcode = SQLAllocHandle(SQL_HANDLE_STMT, sqlconnectionhandle, &sqlstatementhandle);
// Loop through all tables and fetch data
if (retcode == SQL_SUCCESS || retcode == SQL_SUCCESS_WITH_INFO) {
retcode = SQLExecDirect(sqlstatementhandle, (SQLCHAR*)"EXEC sp_MSforeachtable 'SELECT * FROM ?'", SQL_NTS);
// Fetch and print data for each table
while (retcode == SQL_SUCCESS) {
SQLCHAR table_name[SQL_RESULT_LEN];
SQLINTEGER len;
retcode = SQLFetch(sqlstatementhandle);
if (retcode == SQL_SUCCESS || retcode == SQL_SUCCESS_WITH_INFO) {
SQLGetData(sqlstatementhandle, 1, SQL_C_CHAR, table_name, SQL_RESULT_LEN, &len);
cout << "Data for table " << table_name << ":" << endl;
SQLSMALLINT num_cols;
SQLNumResultCols(sqlstatementhandle, &num_cols);
while (SQLFetch(sqlstatementhandle) != SQL_NO_DATA) {
for (int i = 1; i <= num_cols; i++) {
SQLCHAR col_name[SQL_RESULT_LEN];
SQLCHAR col_value[SQL_RESULT_LEN];
SQLINTEGER col_len;
SQLDescribeCol(sqlstatementhandle, i, col_name, SQL_RESULT_LEN, &len, NULL, NULL, NULL, NULL);
SQLGetData(sqlstatementhandle, i, SQL_C_CHAR, col_value, SQL_RESULT_LEN, &col_len);
cout << col_name << ": " << col_value << ", ";
}
cout << endl;
}
}
}
}
}
}
}
}
// Free resources
SQLFreeHandle(SQL_HANDLE_STMT, sqlstatementhandle);
SQLDisconnect(sqlconnectionhandle);
SQLFreeHandle(SQL_HANDLE_DBC, sqlconnectionhandle);
SQLFreeHandle(SQL_HANDLE_ENV, sqlenvhandle);
return 0;
}
```
请注意替换代码中的 "your_server_name"、"your_username" 和 "your_password" 为你的 SQL Server 服务器名称、用户名和密码。
相关推荐
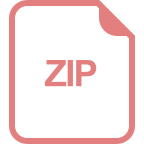
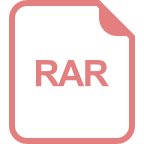















