用python写svm分类问题并可视化
时间: 2023-12-15 19:05:44 浏览: 41
非常感谢您的提问。以下是用Python编写SVM分类问题并可视化的步骤:
1. 导入所需的Python库,包括numpy、pandas、matplotlib和sklearn等。
2. 准备数据: 在这里我们可以使用sklearn的内置数据集Iris,这是一种经典的多类别分类问题数据集。我们通过加载Iris数据集来实现我们的SVM分类模型。
3. 数据预处理:划分数据集为训练数据集和测试数据集。
4. 特征缩放:进行数据标准化以确保归一性。
5. 定义模型并训练:使用sklearn提供的SVM实现,定义一个支持向量机分类器,并在训练数据集上进行训练。
6. 预测结果:对测试集进行预测并计算准确率。
7. 数据可视化:使用matplotlib库绘制分类结果的决策边界,以显示我们的分类器。
下面是示例代码:
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score
# Load Iris dataset
iris = datasets.load_iris()
X = iris.data
y = iris.target
# Split dataset into training and test data sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=0)
# Scaling features to have mean 0 and variance 1
sc = StandardScaler()
sc.fit(X_train)
X_train_std = sc.transform(X_train)
X_test_std = sc.transform(X_test)
# Train SVM classifier on training data
svm = SVC(kernel='linear', C=1.0, random_state=0)
svm.fit(X_train_std, y_train)
# Make predictions using trained classifier
y_pred = svm.predict(X_test_std)
# Calculate model accuracy
accuracy = accuracy_score(y_test, y_pred)
print('Model accuracy:', accuracy)
# Plot decision regions
from matplotlib.colors import ListedColormap
X_combined_std = np.vstack((X_train_std, X_test_std))
y_combined = np.hstack((y_train, y_test))
markers = ('s', 'x', 'o')
colors = ('red', 'blue', 'green')
cmap = ListedColormap(colors[:len(np.unique(y_combined))])
x1_min, x1_max = X_combined_std[:, 0].min() - 1, X_combined_std[:, 0].max() + 1
x2_min, x2_max = X_combined_std[:, 1].min() - 1, X_combined_std[:, 1].max() + 1
xx1, xx2 = np.meshgrid(np.arange(x1_min, x1_max, 0.1), np.arange(x2_min, x2_max, 0.1))
Z = svm.predict(np.array([xx1.ravel(), xx2.ravel()]).T)
Z = Z.reshape(xx1.shape)
plt.contourf(xx1, xx2, Z, alpha=0.3, cmap=cmap)
plt.xlim(xx1.min(), xx1.max())
plt.ylim(xx2.min(), xx2.max())
for idx, cl in enumerate(np.unique(y_combined)):
plt.scatter(x=X_combined_std[y_combined == cl, 0], y=X_combined_std[y_combined == cl, 1],
alpha=0.8, c=cmap(idx),
marker=markers[idx], label=cl)
plt.xlabel('Sepal length [standardized]')
plt.ylabel('Petal length [standardized]')
plt.legend(loc='upper left')
plt.show()
```
希望这个回答对你有所帮助,如果你还有其他问题,请随时问我。
相关推荐
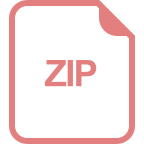
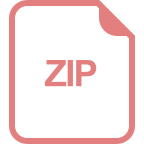
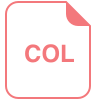
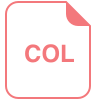
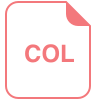
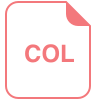
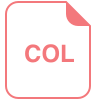









