SDL2.0 渲染 yuv4.0
时间: 2023-10-11 17:12:49 浏览: 110
SDL2.0 支持渲染 YUV4:2:0 格式的视频,需要使用 SDL_Texture 和 SDL_RenderCopy 函数来实现。
以下是一个简单的示例代码:
```c
#include <SDL2/SDL.h>
#include <stdio.h>
const int SCREEN_WIDTH = 640;
const int SCREEN_HEIGHT = 480;
int main(int argc, char* argv[])
{
SDL_Window* window = NULL;
SDL_Renderer* renderer = NULL;
SDL_Texture* texture = NULL;
if (SDL_Init(SDL_INIT_VIDEO) < 0)
{
printf("SDL could not initialize! SDL_Error: %s\n", SDL_GetError());
return 1;
}
window = SDL_CreateWindow("YUV4:2:0 Video", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, SCREEN_WIDTH, SCREEN_HEIGHT, SDL_WINDOW_SHOWN);
if (window == NULL)
{
printf("Window could not be created! SDL_Error: %s\n", SDL_GetError());
return 1;
}
renderer = SDL_CreateRenderer(window, -1, 0);
if (renderer == NULL)
{
printf("Renderer could not be created! SDL_Error: %s\n", SDL_GetError());
return 1;
}
texture = SDL_CreateTexture(renderer, SDL_PIXELFORMAT_YV12, SDL_TEXTUREACCESS_STREAMING, SCREEN_WIDTH, SCREEN_HEIGHT);
if (texture == NULL)
{
printf("Texture could not be created! SDL_Error: %s\n", SDL_GetError());
return 1;
}
// YUV data
Uint8* yPlane = NULL;
Uint8* uPlane = NULL;
Uint8* vPlane = NULL;
int yPitch = 0;
int uPitch = 0;
int vPitch = 0;
int w = 0;
int h = 0;
// read YUV file
FILE* fp = fopen("video.yuv", "rb");
if (fp == NULL)
{
printf("Could not open YUV file!\n");
return 1;
}
// allocate memory for YUV data
int bufferSize = SCREEN_WIDTH * SCREEN_HEIGHT * 3 / 2;
Uint8* buffer = (Uint8*)malloc(bufferSize);
// main loop
SDL_Event event;
bool quit = false;
while (!quit)
{
while (SDL_PollEvent(&event))
{
if (event.type == SDL_QUIT)
{
quit = true;
}
}
// read YUV data
if (fread(buffer, 1, bufferSize, fp) != bufferSize)
{
// end of file
rewind(fp);
}
// set YUV data
yPlane = buffer;
uPlane = yPlane + SCREEN_WIDTH * SCREEN_HEIGHT;
vPlane = uPlane + SCREEN_WIDTH * SCREEN_HEIGHT / 4;
yPitch = SCREEN_WIDTH;
uPitch = SCREEN_WIDTH / 2;
vPitch = SCREEN_WIDTH / 2;
w = SCREEN_WIDTH;
h = SCREEN_HEIGHT;
// update texture
SDL_UpdateYUVTexture(texture, NULL, yPlane, yPitch, uPlane, uPitch, vPlane, vPitch);
// clear screen
SDL_SetRenderDrawColor(renderer, 0xFF, 0xFF, 0xFF, 0xFF);
SDL_RenderClear(renderer);
// render texture
SDL_RenderCopy(renderer, texture, NULL, NULL);
// update screen
SDL_RenderPresent(renderer);
}
// free memory
free(buffer);
// cleanup
SDL_DestroyTexture(texture);
SDL_DestroyRenderer(renderer);
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
```
在代码中,我们首先创建一个 SDL 窗口和渲染器。然后,使用 SDL_CreateTexture 函数创建一个 YV12 格式的纹理。接下来,读取 YUV 文件,并将数据设置到纹理中。在主循环中,使用 SDL_RenderCopy 函数将纹理渲染到屏幕上。最后,记得在程序结束前释放所有内存和资源。
阅读全文
相关推荐
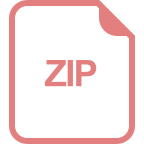
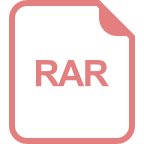
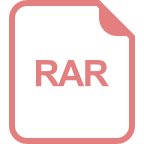
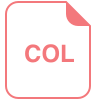
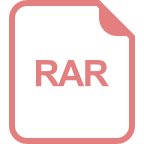
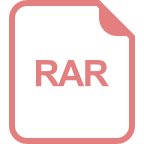
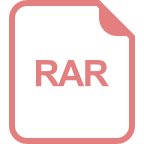
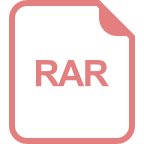
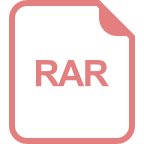
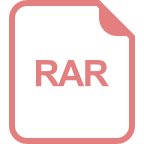
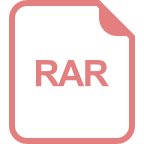
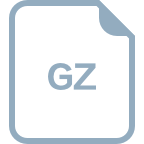
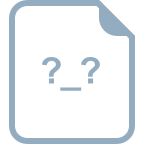
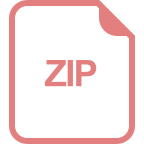
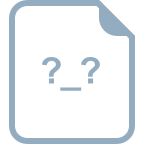
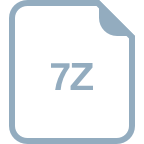
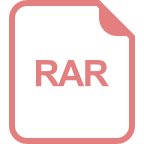
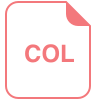
