用vue+Springboot写一个登录界面
时间: 2024-05-02 19:16:52 浏览: 112
好的,下面是一个简单的登录界面的代码示例,使用Vue.js作为前端框架,Spring Boot作为后端框架,使用RESTful API进行数据交互。
前端代码示例:
```
<template>
<div>
<h2>Login</h2>
<form>
<div>
<label>Username:</label>
<input type="text" v-model="username">
</div>
<div>
<label>Password:</label>
<input type="password" v-model="password">
</div>
<div>
<button type="submit" v-on:click.prevent="login">Login</button>
</div>
</form>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
username: '',
password: ''
}
},
methods: {
login() {
axios.post('/api/login', {
username: this.username,
password: this.password
})
.then(response => {
console.log(response);
// do something after successful login
})
.catch(error => {
console.log(error);
// handle login error
});
}
}
}
</script>
```
后端代码示例:
```
@RestController
@RequestMapping("/api")
public class LoginController {
@PostMapping("/login")
public ResponseEntity<?> login(@RequestBody LoginRequest loginRequest) {
// perform login authentication
// return JWT token if successful
}
// other controller methods
}
public class LoginRequest {
private String username;
private String password;
// getters and setters
}
```
其中,前端代码使用Vue.js框架进行实现,使用axios库进行RESTful API调用。后端代码使用Spring Boot框架进行实现,使用@RestController注释声明RESTful API,使用@PostMapping注释声明POST请求,使用@RequestBody注释声明请求体,使用ResponseEntity<?>作为返回类型,其中?表示任意类型。此外,LoginRequest类用于接收前端POST请求中的请求体数据。
阅读全文
相关推荐
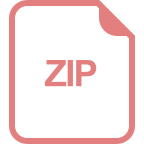
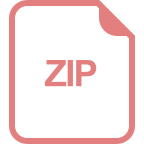
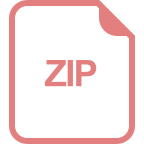









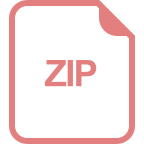
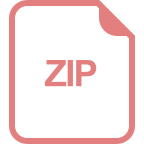
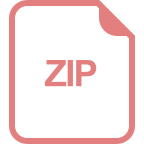
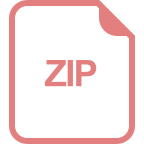
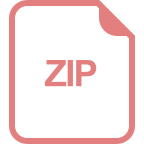
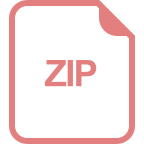
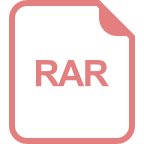