C++中如何确定string字符串的字符数
时间: 2023-10-16 12:09:53 浏览: 36
可以使用string类的size()或length()方法来确定字符串的字符数。例如,以下代码可以输出字符串s中的字符数:
```
#include <iostream>
#include <string>
using namespace std;
int main() {
string s = "Hello World";
int len = s.size(); // 或者 s.length();
cout << "字符串s中的字符数为:" << len << endl;
return 0;
}
```
输出结果为:
```
字符串s中的字符数为:11
```
相关问题
c++中遍历string字符串中文乱码
在 C++ 中,字符串默认是使用 ASCII 编码的,而中文字符使用的是多字节编码,例如 UTF-8 等。因此,如果直接遍历字符串的每一个字符,可能会出现乱码的情况。
解决方法是使用迭代器来遍历字符串,因为迭代器会按照字符的实际长度来移动,而不是按照字节长度来移动。
下面是一个示例代码:
```
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str = "你好,世界!";
for (auto it = str.begin(); it != str.end(); ++it)
{
cout << *it;
}
cout << endl;
return 0;
}
```
输出结果为:
```
你好,世界!
```
使用迭代器遍历字符串时,不需要担心中文字符的长度问题,因为迭代器会自动处理。
c++中按字符数分割string类型字符串
你可以使用STL中的`std::string`和`std::vector`来实现按字符数分割`string`类型字符串,具体步骤如下:
1. 定义一个`std::string`类型的字符串变量,用于存放要分割的字符串。
2. 定义一个整数变量,用于指定每个子字符串的字符数。
3. 定义一个`std::vector<std::string>`类型的变量,用于存放分割后的子字符串。
4. 使用循环遍历整个字符串,每隔指定的字符数将字符串截取成一个子字符串,并将其添加到`std::vector`容器中。
下面是一个示例代码:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
vector<string> splitString(const string& str, int len) {
vector<string> result;
for (int i = 0; i < str.size(); i += len) {
result.push_back(str.substr(i, len));
}
return result;
}
int main() {
string str = "Hello, world! This is a test string.";
int len = 5;
vector<string> parts = splitString(str, len);
for (auto& part : parts) {
cout << part << endl;
}
return 0;
}
```
这个示例代码可以将字符串按照每5个字符进行分割。`splitString`函数接受两个参数,第一个参数是要分割的字符串,第二个参数是每个子字符串的字符数。函数返回一个`std::vector<std::string>`类型的容器,其中存放了分割后的子字符串。在`main`函数中,我们将一个字符串分割成长度为5的子字符串,并打印出来。
相关推荐
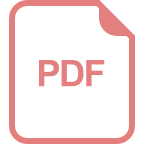
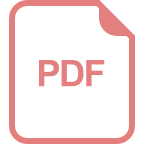
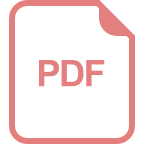
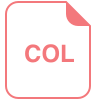
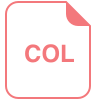
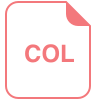
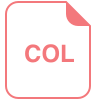
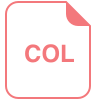







