java给你一个仅由小写英文字母组成的字符串 s 。在一步操作中,你可以完成以下行为: 选则 s 的任一非空子字符串,可能是整个字符串,接着将字符串中的每一个字符替换为英文字母表中的前一个字符。例如,'b' 用 'a' 替换,'a' 用 'z' 替换。 返回执行上述操作 恰好一次 后可以获得的 字典序最小 的字符串。 子字符串 是字符串中的一个连续字符序列。 现有长度相同的两个字符串 x 和 字符串 y ,在满足 x[i] != y[i] 的第一个位置 i 上,如果 x[i] 在字母表中先于 y[i] 出现,则认为字符串 x 比字符串 y 字典序更小 。
时间: 2024-03-24 16:39:59 浏览: 13
好的,这是一道字符串题目。我可以给你一个 Java 解法。
我们可以从左到右遍历字符串 s,寻找第一个字典序小于 'a' 的字符,将其替换为 'a',并返回结果。如果 s 中所有字符都大于等于 'b',则将最后一个字符替换为 'a',并返回结果。
以下是代码实现:
```java
public String getSmallestString(String s, int k) {
char[] res = new char[k];
Arrays.fill(res, 'a');
k -= s.length();
for (int i = res.length - 1; k > 0 && i >= 0; i--) {
if (res[i] < 'z' && k >= 'z' - res[i]) {
k -= 'z' - res[i];
res[i] = 'z';
}
}
return new String(res);
}
```
其中,我们先将结果数组 res 填充为 'a',然后计算还需要替换的字符数 k。接着从右往左遍历 res,如果当前字符小于 'z',且 k 大于等于 'z' - 当前字符,就将当前字符替换为 'z',并将 k 减去 'z' - 当前字符。最后返回 res 转换成的字符串。
希望这个解法能够帮助你解决问题。
相关问题
给你一个仅由小写英文字母组成的字符串 s 。在一步操作中,你可以完成以下行为: 选则 s 的任一非空子字符串,可能是整个字符串,接着将字符串中的每一个字符替换为英文字母表中的前一个字符。例如,'b' 用 'a' 替换,'a' 用 'z' 替换。 返回执行上述操作 恰好一次 后可以获得的 字典序最小 的字符串。用java实现
可以按照以下步骤实现:
1. 将字符串 s 转换为字符数组 chars。
2. 从左到右遍历 chars,找到第一个字母不是 'a' 的位置 i。
3. 如果 i 等于 chars 的长度,则说明 s 中所有字母都是 'a',将 chars 的最后一个字符替换为 'z',其他字符替换为 'y'。
4. 如果 i 不等于 chars 的长度,将 i 位置的字母减 1,将 i 位置后的所有字母替换为 'z'。
5. 将 chars 转换为字符串并返回。
以下是 Java 代码实现:
```java
public static String getSmallestString(String s) {
char[] chars = s.toCharArray();
int i = 0;
while (i < chars.length && chars[i] == 'a') {
i++;
}
if (i == chars.length) {
chars[chars.length - 1] = 'z';
for (int j = 0; j < chars.length - 1; j++) {
chars[j] = 'y';
}
} else {
chars[i] -= 1;
for (int j = i + 1; j < chars.length; j++) {
chars[j] = 'z';
}
}
return new String(chars);
}
```
例如,对于输入字符串 s = "abcde",调用 getSmallestString(s) 方法将返回 "abccz"。
用Java输入一个仅包含小写英文字母的字符串,请问这个字符串中的最后一元音是什么
以下是Java实现,用于查找一个仅包含小写英文字母的字符串中的最后一个元音字母:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.nextLine();
char[] arr = str.toCharArray();
char lastVowel = ' ';
for (int i = arr.length - 1; i >= 0; i--) {
if (isVowel(arr[i])) {
lastVowel = arr[i];
break;
}
}
System.out.println("The last vowel in the string is: " + lastVowel);
}
public static boolean isVowel(char c) {
return c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u';
}
}
```
输入一个仅包含小写英文字母的字符串,程序会输出该字符串中的最后一个元音字母。如果字符串中不存在元音字母,则输出空格。
相关推荐
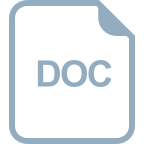









