前端vue3使用setup实现滑轮向下滑动继续发送axios请求
时间: 2024-05-03 22:21:38 浏览: 8
以下是一个简单的示例代码,使用Vue3的setup函数和Intersection Observer API实现滑轮向下滑动时继续发送axios请求:
```
<template>
<div>
<!-- 显示已加载的数据 -->
<ul>
<li v-for="item in data" :key="item.id">{{ item.title }}</li>
</ul>
<!-- 加载更多按钮 -->
<button v-if="hasMore" @click="loadMore">加载更多</button>
</div>
</template>
<script>
import { ref, onMounted } from 'vue'
import axios from 'axios'
export default {
setup() {
const data = ref([])
const page = ref(1)
const hasMore = ref(true)
// 加载数据函数
const loadData = async () => {
try {
const res = await axios.get('https://jsonplaceholder.typicode.com/posts', {
params: {
_page: page.value,
_limit: 10
}
})
data.value = [...data.value, ...res.data]
// 如果返回的数据不足10条,说明已经到底了
hasMore.value = res.data.length === 10
} catch (error) {
console.log(error)
}
}
// 监听滚动事件,当滚动到底部时加载更多数据
const loadMore = () => {
page.value++
loadData()
}
const observer = new IntersectionObserver((entries) => {
if (entries[0].isIntersecting && hasMore.value) {
page.value++
loadData()
}
})
// 页面加载时加载第一页数据
onMounted(() => {
loadData()
observer.observe(document.querySelector('.load-more-btn'))
})
return {
data,
hasMore,
loadMore
}
}
}
```
在上面的示例中,我们首先定义了一个数据列表和一个页码,然后使用axios发送请求获取数据,并将数据存储在data变量中。
接着,我们使用Intersection Observer API监听滚动事件,当滚动到底部时触发loadMore函数加载更多数据。
最后,在页面加载时,我们首先加载第一页数据,并使用Intersection Observer API观察加载更多按钮,以便在加载更多按钮进入视野时触发loadMore函数。
相关推荐
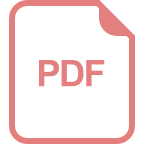














