怎样用opencv编写身份证扫描合并到一张图片
时间: 2024-02-28 08:56:50 浏览: 32
首先,你需要安装OpenCV库。然后,你可以使用以下步骤来编写身份证扫描合并到一张图片:
1. 读取身份证图像和背景图像。
2. 对身份证图像进行灰度化和二值化处理,使其变成黑白图像。
3. 使用轮廓检测算法(如findContours函数)找到身份证图像的轮廓。
4. 对轮廓进行筛选,只保留符合要求的轮廓(如形状、大小等)。
5. 使用透视变换(如warpPerspective函数)将身份证图像变换到目标区域。
6. 将变换后的身份证图像与背景图像合并到一张图片上。
下面是一个示例代码:
```python
import cv2
# 读取身份证图像和背景图像
id_card_img = cv2.imread("id_card.jpg")
bg_img = cv2.imread("bg.jpg")
# 对身份证图像进行灰度化和二值化处理
gray = cv2.cvtColor(id_card_img, cv2.COLOR_BGR2GRAY)
ret, binary = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV | cv2.THRESH_OTSU)
# 找到身份证图像的轮廓
contours, hierarchy = cv2.findContours(binary, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 筛选符合要求的轮廓
for contour in contours:
if cv2.contourArea(contour) > 10000:
x, y, w, h = cv2.boundingRect(contour)
if w > 200 and h > 200:
# 使用透视变换将身份证图像变换到目标区域
src_points = np.array([[x, y], [x + w, y], [x + w, y + h], [x, y + h]], dtype=np.float32)
dst_points = np.array([[500, 500], [1000, 500], [1000, 1000], [500, 1000]], dtype=np.float32)
M = cv2.getPerspectiveTransform(src_points, dst_points)
id_card_img_transformed = cv2.warpPerspective(id_card_img, M, (1500, 1500))
break
# 将变换后的身份证图像与背景图像合并到一张图片上
bg_img[500:2000, 500:2000] = id_card_img_transformed
cv2.imshow("merged image", bg_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请注意,上述代码仅供参考,具体实现可能需要根据实际情况进行调整。
相关推荐
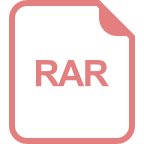
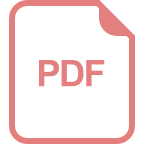
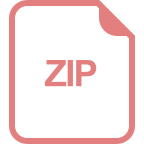
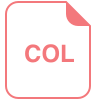
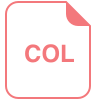
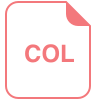
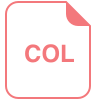
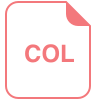









