遗传算法python实例
时间: 2023-10-26 19:08:13 浏览: 46
以下是一个简单的遗传算法 Python 实例:
```python
import random
# 定义问题
problem = [1, 3, 5, 7, 9, 11, 13, 15]
# 定义每个个体的长度
gene_length = len(problem)
# 定义种群大小
population_size = 50
# 定义迭代次数
generation = 100
# 定义交叉概率
crossover_probability = 0.9
# 定义变异概率
mutation_probability = 0.05
# 定义选择操作
def selection(population):
# 计算每个个体的适应度
fitness = []
for individual in population:
fitness.append(sum(individual))
# 选择最小的适应度
min_fitness = min(fitness)
# 计算每个个体的选择概率
selection_probability = []
for i in range(len(population)):
selection_probability.append((min_fitness + 1) / (fitness[i] + 1))
# 根据选择概率随机选择两个个体
index1 = random.choices(range(len(population)), weights=selection_probability)[0]
index2 = random.choices(range(len(population)), weights=selection_probability)[0]
return population[index1], population[index2]
# 定义交叉操作
def crossover(parent1, parent2):
# 随机选择一个交叉点
crossover_point = random.randint(1, gene_length - 1)
# 交叉生成两个子代
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
# 定义变异操作
def mutation(individual):
# 随机选择一个基因进行变异
mutation_point = random.randint(0, gene_length - 1)
# 将该基因随机变为另一个值
individual[mutation_point] = random.choice(problem)
return individual
# 初始化种群
population = []
for i in range(population_size):
population.append([random.choice(problem) for j in range(gene_length)])
# 进化
for i in range(generation):
# 计算每个个体的适应度
fitness = []
for individual in population:
fitness.append(sum(individual))
# 打印最优解
print('Generation:', i, 'Best Solution:', population[fitness.index(min(fitness))], 'Fitness:', min(fitness))
# 生成下一代种群
new_population = []
for j in range(population_size // 2):
# 选择两个个体
parent1, parent2 = selection(population)
# 判断是否进行交叉
if random.random() < crossover_probability:
child1, child2 = crossover(parent1, parent2)
else:
child1, child2 = parent1, parent2
# 判断是否进行变异
if random.random() < mutation_probability:
child1 = mutation(child1)
if random.random() < mutation_probability:
child2 = mutation(child2)
# 将子代加入新种群
new_population.append(child1)
new_population.append(child2)
population = new_population
```
以上的例子是一个求解八皇后问题的遗传算法,其中 `problem` 定义了每个皇后所在的列数,`gene_length` 定义了每个个体的长度,`population_size` 定义了种群大小,`generation` 定义了迭代次数,`crossover_probability` 定义了交叉概率,`mutation_probability` 定义了变异概率。
在代码中,首先通过随机生成的方式初始化了种群,然后进行了多次进化,每次进化都会选择出两个个体进行交叉和变异,并将生成的子代加入新种群。在选择个体时,使用了轮盘赌选择算法来计算每个个体的选择概率,并根据概率随机选择两个个体。在交叉操作中,随机选择了一个交叉点,并将两个个体在交叉点处进行了交叉。在变异操作中,随机选择了一个基因进行变异,并将该基因随机变为另一个值。
最后,打印出了每一代种群中的最优解和适应度。
相关推荐
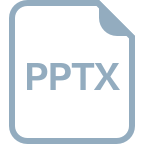
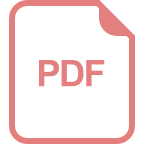
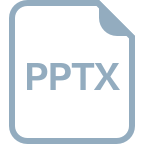