属性约简算法的设计与实现MATLAB代码
时间: 2023-10-28 17:06:07 浏览: 36
属性约简算法是一种数据挖掘方法,通常用于降低数据维度,去除冗余信息,同时保留最重要的特征。在MATLAB中,可以使用以下代码实现属性约简算法:
1. 导入数据
```matlab
data = load('data.txt'); % data.txt为数据文件
X = data(:, 1:end-1); % 特征矩阵
Y = data(:, end); % 标签矩阵
```
2. 计算信息熵和条件熵
```matlab
% 计算信息熵
function [entropy] = entropy(Y)
classes = unique(Y);
entropy = 0;
for i=1:length(classes)
p = sum(Y==classes(i)) / length(Y);
entropy = entropy - p*log2(p);
end
end
% 计算条件熵
function [cond_entropy] = cond_entropy(X, Y, feature)
classes = unique(Y);
values = unique(X(:, feature));
cond_entropy = 0;
for i=1:length(values)
p = sum(X(:, feature)==values(i)) / length(X(:, feature));
for j=1:length(classes)
pj = sum(X(:, feature)==values(i) & Y==classes(j)) / sum(X(:, feature)==values(i));
if pj ~= 0
cond_entropy = cond_entropy - p*pj*log2(pj);
end
end
end
end
```
3. 计算信息增益
```matlab
function [gain] = info_gain(X, Y, feature)
entropy_Y = entropy(Y);
cond_entropy_X_Y = cond_entropy(X, Y, feature);
gain = entropy_Y - cond_entropy_X_Y;
end
```
4. 属性约简算法
```matlab
function [reduced_features] = attribute_reduction(X, Y)
n_features = size(X, 2);
C = 1:n_features; % 候选属性集合
D = []; % 约简后的属性集合
while ~isempty(C)
max_gain = -inf;
max_feature = 0;
for i=1:length(C)
gain = info_gain(X, Y, C(i));
if gain > max_gain
max_gain = gain;
max_feature = C(i);
end
end
D = [D max_feature];
C = setdiff(C, max_feature);
for i=1:length(C)
if ~isempty(intersect(D, C(i)))
C(i) = [];
end
end
end
reduced_features = D;
end
```
5. 测试
```matlab
reduced_features = attribute_reduction(X, Y);
fprintf('Reduced features: %s\n', num2str(reduced_features));
```
这是一个简单的属性约简算法的实现,实际应用中还需要考虑更多的因素,如特征选择的方法、数据预处理等。
相关推荐
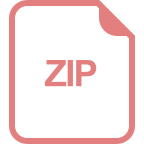














