python编写GUI目标检测系统程序的代码
时间: 2024-01-13 13:05:45 浏览: 32
以下是一个简单的Python GUI目标检测系统程序的代码示例,使用了OpenCV和Tkinter库:
```python
import cv2
import tkinter as tk
from tkinter import filedialog
class ObjectDetector:
def __init__(self):
self.image_path = ''
self.classifier_path = 'cascade.xml'
self.scale_factor = 1.1
self.min_neighbors = 5
self.min_size = (30, 30)
self.window = tk.Tk()
self.window.title('Object Detector')
self.window.geometry('400x300')
self.canvas = tk.Canvas(self.window, width=400, height=250)
self.canvas.pack()
self.btn_browse = tk.Button(self.window, text='Browse', command=self.browse_file)
self.btn_browse.pack(pady=10)
self.btn_detect = tk.Button(self.window, text='Detect', command=self.detect_objects)
self.btn_detect.pack(pady=10)
def browse_file(self):
self.image_path = filedialog.askopenfilename(initialdir='/', title='Select Image File', filetypes=(('JPEG Files', '*.jpg'), ('PNG Files', '*.png'), ('All Files', '*.*')))
img = cv2.imread(self.image_path)
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
height, width, channels = img.shape
self.canvas.config(width=width, height=height)
self.photo = tk.PhotoImage(width=width, height=height)
self.photo.blank()
self.photo = self.convert_img_to_photo(img)
self.canvas.create_image(0, 0, anchor=tk.NW, image=self.photo)
def detect_objects(self):
if self.image_path == '':
tk.messagebox.showerror('Error', 'Please select an image file.')
return
img = cv2.imread(self.image_path)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
classifier = cv2.CascadeClassifier(self.classifier_path)
objects = classifier.detectMultiScale(gray, scaleFactor=self.scale_factor, minNeighbors=self.min_neighbors, minSize=self.min_size)
for (x, y, w, h) in objects:
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
self.photo = self.convert_img_to_photo(img)
self.canvas.create_image(0, 0, anchor=tk.NW, image=self.photo)
def convert_img_to_photo(self, img):
height, width, channels = img.shape
photo = tk.PhotoImage(width=width, height=height)
photo.blank()
photo = self.put_img_into_photo(img, photo, height, width)
return photo
def put_img_into_photo(self, img, photo, height, width):
for y in range(height):
for x in range(width):
r, g, b = img[y][x]
color = '#{:02x}{:02x}{:02x}'.format(r, g, b)
photo.put(color, (x, y))
return photo
def run(self):
self.window.mainloop()
if __name__ == '__main__':
detector = ObjectDetector()
detector.run()
```
该程序包括一个GUI窗口,允许用户通过文件选择对话框选择要检测的图像文件。然后使用OpenCV库中的CascadeClassifier类来检测图像中的对象,并将其绘制在图像中。最后,将绘制后的图像显示在GUI窗口中。
相关推荐
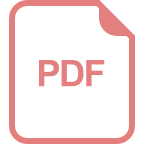















