使用pyside2实现没有signal的线程池
时间: 2023-05-17 22:04:18 浏览: 228
可以使用Python内置的concurrent.futures模块来实现线程池,而不需要使用signal。以下是一个使用pyside2和concurrent.futures模块实现线程池的示例代码:
```python
from PySide2.QtCore import QObject, QThreadPool, QRunnable, Slot
import concurrent.futures
class Worker(QRunnable):
def __init__(self, func, *args, **kwargs):
super().__init__()
self.func = func
self.args = args
self.kwargs = kwargs
@Slot()
def run(self):
self.func(*self.args, **self.kwargs)
class ThreadPool(QObject):
def __init__(self, max_threads=5):
super().__init__()
self.threadpool = QThreadPool.globalInstance()
self.executor = concurrent.futures.ThreadPoolExecutor(max_workers=max_threads)
def submit(self, func, *args, **kwargs):
worker = Worker(func, *args, **kwargs)
self.threadpool.start(worker)
def submit_blocking(self, func, *args, **kwargs):
future = self.executor.submit(func, *args, **kwargs)
return future.result()
```
使用示例:
```python
def my_func(arg1, arg2):
print(arg1, arg2)
pool = ThreadPool()
pool.submit(my_func, "hello", "world")
```
这将在线程池中启动一个新线程来运行my_func函数,传递参数"hello"和"world"。如果您需要等待函数执行完成并获取返回值,可以使用submit_blocking方法。
阅读全文
相关推荐










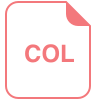



