告诉我使用ASP.NET做一个界面美丽的登陆网页的前端和后端代码
时间: 2023-06-24 22:09:01 浏览: 129
以下是使用 ASP.NET 实现一个美丽的登录页面的前端和后端代码:
前端代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>美丽的登录页面</title>
<link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet" />
<link href="https://fonts.googleapis.com/css?family=Open+Sans:400,600" rel="stylesheet" />
<style>
body {
background: #f1f1f1;
font-family: 'Open Sans', sans-serif;
}
.login-form {
background-color: #ffffff;
border-radius: 5px;
box-shadow: 0px 0px 10px 1px rgba(0,0,0,0.2);
margin-top: 50px;
padding: 25px;
}
.login-form .form-control {
border-radius: 0;
border: none;
border-bottom: 1px solid #ddd;
box-shadow: none;
outline: none;
}
.login-form .form-group {
margin-bottom: 20px;
}
.login-form h2 {
margin-bottom: 25px;
text-align: center;
}
.login-form button {
background-color: #2ecc71;
border: none;
border-radius: 0;
box-shadow: none;
color: #ffffff;
padding: 10px 20px;
text-transform: uppercase;
transition: all 0.3s ease 0s;
width: 100%;
}
.login-form button:hover {
background-color: #27ae60;
}
</style>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-4 col-md-offset-4">
<div class="login-form">
<h2>登录</h2>
<form action="Login.aspx" method="post">
<div class="form-group">
<input type="text" name="username" placeholder="用户名" class="form-control" required />
</div>
<div class="form-group">
<input type="password" name="password" placeholder="密码" class="form-control" required />
</div>
<div class="form-group">
<button type="submit">登录</button>
</div>
</form>
</div>
</div>
</div>
</div>
</body>
</html>
```
后端代码:
```csharp
using System;
using System.Data.SqlClient;
public partial class Login : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnLogin_Click(object sender, EventArgs e)
{
string username = txtUsername.Text.Trim();
string password = txtPassword.Text.Trim();
string connectionString = "Data Source=(local);Initial Catalog=MyDatabase;Integrated Security=True";
SqlConnection connection = new SqlConnection(connectionString);
string sql = "SELECT COUNT(*) FROM Users WHERE Username=@username AND Password=@password";
SqlCommand command = new SqlCommand(sql, connection);
command.Parameters.AddWithValue("@username", username);
command.Parameters.AddWithValue("@password", password);
connection.Open();
int count = (int)command.ExecuteScalar();
connection.Close();
if (count > 0)
{
Response.Redirect("Welcome.aspx");
}
else
{
lblMessage.Text = "用户名或密码错误!";
}
}
}
```
这里的 `Login.aspx` 页面和 `Welcome.aspx` 页面需要自己根据实际情况编写。
阅读全文
相关推荐
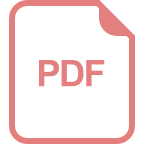
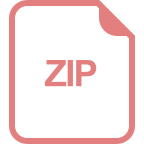
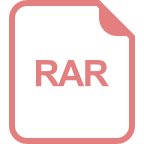
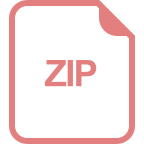
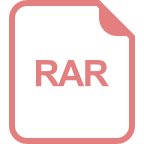
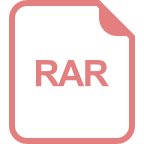
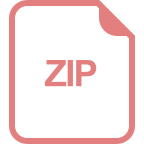
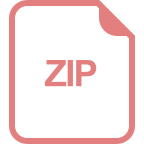
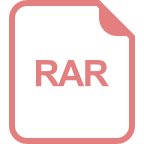
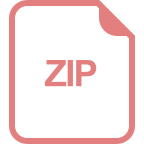
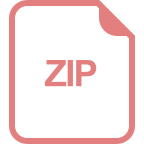
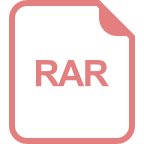
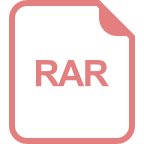
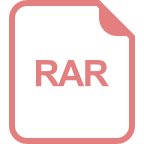
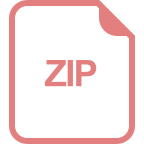
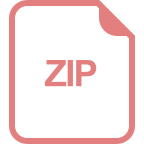