c语言俄罗斯方块代码
时间: 2023-08-12 09:17:15 浏览: 112
以下是一个简单的C语言俄罗斯方块代码示例:
```c
#include <stdio.h>
#include <conio.h>
#include <stdlib.h>
#include <time.h>
#include <windows.h>
#define WIDTH 12 // 画面宽度
#define HEIGHT 22 // 画面高度
#define BLOCK_SIZE 4 // 方块大小
#define BLOCK_NUM 7 // 方块数量
int block[BLOCK_NUM][BLOCK_SIZE][BLOCK_SIZE] = {
// I
{
{0, 0, 0, 0},
{1, 1, 1, 1},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// J
{
{1, 0, 0},
{1, 1, 1},
{0, 0, 0}
},
// L
{
{0, 0, 1},
{1, 1, 1},
{0, 0, 0}
},
// O
{
{1, 1},
{1, 1}
},
// S
{
{0, 1, 1},
{1, 1, 0},
{0, 0, 0}
},
// T
{
{0, 1, 0},
{1, 1, 1},
{0, 0, 0}
},
// Z
{
{1, 1, 0},
{0, 1, 1},
{0, 0, 0}
}
};
int screen[HEIGHT][WIDTH] = {0}; // 画面
int x, y; // 当前方块位置
int blockType; // 当前方块类型
int blockRotation; // 当前方块旋转状态
int score; // 得分
void drawBlock(int x, int y, int blockType, int blockRotation); // 绘制方块
void clearScreen(); // 清空画面
void showScreen(); // 显示画面
int checkCollision(int x, int y, int blockType, int blockRotation); // 检查碰撞
void placeBlock(int x, int y, int blockType, int blockRotation); // 放置方块
void clearLines(); // 消行
void gameOver(); // 游戏结束
int main() {
int key;
srand(time(NULL));
blockType = rand() % BLOCK_NUM;
blockRotation = rand() % 4;
x = WIDTH / 2 - 2;
y = 0;
while (1) {
// 获取用户输入
if (kbhit()) {
key = getch();
switch (key) {
case 'a':
if (!checkCollision(x - 1, y, blockType, blockRotation)) {
x--;
}
break;
case 'd':
if (!checkCollision(x + 1, y, blockType, blockRotation)) {
x++;
}
break;
case 's':
if (!checkCollision(x, y + 1, blockType, blockRotation)) {
y++;
}
break;
case 'w':
if (!checkCollision(x, y, blockType, (blockRotation + 1) % 4)) {
blockRotation = (blockRotation + 1) % 4;
}
break;
case ' ':
while (!checkCollision(x, y + 1, blockType, blockRotation)) {
y++;
}
break;
}
}
// 判断方块是否落地
if (checkCollision(x, y + 1, blockType, blockRotation)) {
placeBlock(x, y, blockType, blockRotation);
int lines = clearLines();
score += lines * lines;
if (y == 0) {
gameOver();
return 0;
}
x = WIDTH / 2 - 2;
y = 0;
blockType = rand() % BLOCK_NUM;
blockRotation = rand() % 4;
}
// 绘制画面
clearScreen();
drawBlock(x, y, blockType, blockRotation);
showScreen();
Sleep(50);
}
return 0;
}
// 绘制方块
void drawBlock(int x, int y, int blockType, int blockRotation) {
int i, j;
for (i = 0; i < BLOCK_SIZE; i++) {
for (j = 0; j < BLOCK_SIZE; j++) {
if (block[blockType][i][j]) {
screen[y + i][x + j] = blockType + 1;
}
}
}
}
// 清空画面
void clearScreen() {
memset(screen, 0, sizeof(screen));
}
// 显示画面
void showScreen() {
int i, j;
for (i = 0; i < HEIGHT; i++) {
for (j = 0; j < WIDTH; j++) {
switch (screen[i][j]) {
case 0:
printf(" ");
break;
case 1:
printf("I");
break;
case 2:
printf("J");
break;
case 3:
printf("L");
break;
case 4:
printf("O");
break;
case 5:
printf("S");
break;
case 6:
printf("T");
break;
case 7:
printf("Z");
break;
}
}
printf("\n");
}
printf("Score: %d\n", score);
}
// 检查碰撞
int checkCollision(int x, int y, int blockType, int blockRotation) {
int i, j;
for (i = 0; i < BLOCK_SIZE; i++) {
for (j = 0; j < BLOCK_SIZE; j++) {
if (block[blockType][i][j]) {
int screenX = x + j;
int screenY = y + i;
if (screenX < 0 || screenX >= WIDTH || screenY < 0 || screenY >= HEIGHT || screen[screenY][screenX]) {
return 1;
}
}
}
}
return 0;
}
// 放置方块
void placeBlock(int x, int y, int blockType, int blockRotation) {
int i, j;
for (i = 0; i < BLOCK_SIZE; i++) {
for (j = 0; j < BLOCK_SIZE; j++) {
if (block[blockType][i][j]) {
screen[y + i][x + j] = blockType + 1;
}
}
}
}
// 消行
void clearLines() {
int i, j, k;
int lines = 0;
for (i = HEIGHT - 1; i >= 0; i--) {
int isLine = 1;
for (j = 0; j < WIDTH; j++) {
if (!screen[i][j]) {
isLine = 0;
break;
}
}
if (isLine) {
lines++;
for (k = i; k > 0; k--) {
memcpy(screen[k], screen[k - 1], sizeof(int) * WIDTH);
}
memset(screen[0], 0, sizeof(int) * WIDTH);
i++;
}
}
return lines;
}
// 游戏结束
void gameOver() {
printf("Game Over!\n");
printf("Score: %d\n", score);
}
```
以上代码实现了一个简单的俄罗斯方块游戏,使用了控制台窗口作为画面,通过键盘输入控制方块移动和旋转。
阅读全文
相关推荐
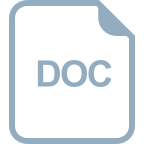
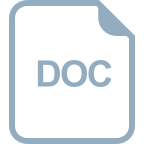
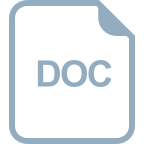
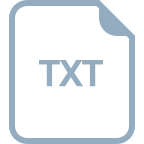
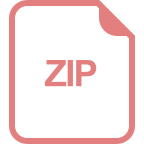
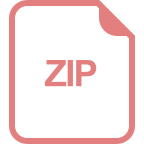
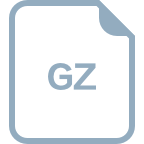
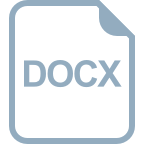
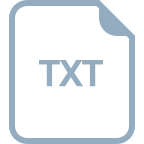
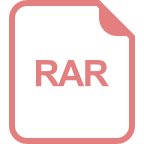
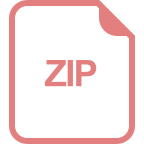
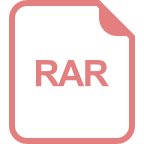

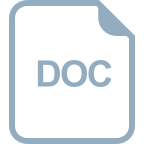
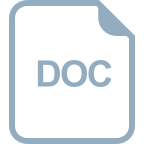
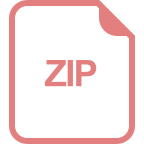
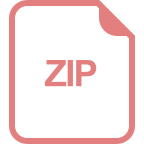
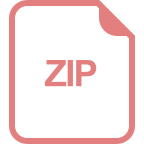