安卓中扫描特定类型的外围BLE蓝牙设备获取列表完整详细的用例
时间: 2024-03-12 19:44:07 浏览: 112
好的,您可以参考以下的安卓代码,它演示了如何扫描特定类型的外围BLE蓝牙设备并获取列表的完整用例。
```java
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
import android.bluetooth.BluetoothGatt;
import android.bluetooth.BluetoothGattCallback;
import android.bluetooth.BluetoothGattCharacteristic;
import android.bluetooth.BluetoothGattDescriptor;
import android.bluetooth.BluetoothGattService;
import android.bluetooth.BluetoothManager;
import android.bluetooth.le.BluetoothLeScanner;
import android.bluetooth.le.ScanCallback;
import android.bluetooth.le.ScanResult;
import android.bluetooth.le.ScanSettings;
import android.content.Context;
import android.os.Handler;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
public class BleScanner {
private static final String TAG = "BleScanner";
private static final long SCAN_PERIOD = 10000; // 扫描时间,单位毫秒
private BluetoothAdapter mBluetoothAdapter;
private BluetoothLeScanner mBluetoothLeScanner;
private ScanCallback mScanCallback;
private Handler mHandler;
private boolean mScanning;
private List<BluetoothDevice> mDeviceList;
private Context mContext;
public BleScanner(Context context) {
mContext = context;
mHandler = new Handler();
BluetoothManager bluetoothManager = (BluetoothManager) context.getSystemService(Context.BLUETOOTH_SERVICE);
mBluetoothAdapter = bluetoothManager.getAdapter();
mBluetoothLeScanner = mBluetoothAdapter.getBluetoothLeScanner();
mDeviceList = new ArrayList<>();
}
public void startScan(UUID serviceUuid) {
if (mScanning) {
return;
}
ScanSettings settings = new ScanSettings.Builder().setScanMode(ScanSettings.SCAN_MODE_LOW_LATENCY).build();
mScanCallback = new ScanCallback() {
@Override
public void onScanResult(int callbackType, ScanResult result) {
BluetoothDevice device = result.getDevice();
if (device != null && device.getName() != null && device.getName().contains("MY_DEVICE_NAME")) {
BluetoothGatt gatt = device.connectGatt(mContext, false, mGattCallback);
gatt.discoverServices();
}
}
};
mBluetoothLeScanner.startScan(null, settings, mScanCallback);
mScanning = true;
mHandler.postDelayed(() -> stopScan(), SCAN_PERIOD);
}
public void stopScan() {
if (mScanning && mBluetoothAdapter != null && mBluetoothLeScanner != null) {
mBluetoothLeScanner.stopScan(mScanCallback);
mScanCallback = null;
mScanning = false;
}
}
private BluetoothGattCallback mGattCallback = new BluetoothGattCallback() {
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
if (newState == BluetoothGatt.STATE_CONNECTED) {
gatt.discoverServices();
} else if (newState == BluetoothGatt.STATE_DISCONNECTED) {
gatt.close();
}
}
@Override
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
if (status == BluetoothGatt.GATT_SUCCESS) {
for (BluetoothGattService service : gatt.getServices()) {
if (service.getUuid().equals(UUID.fromString("MY_SERVICE_UUID"))) {
mDeviceList.add(gatt.getDevice());
}
}
gatt.disconnect();
}
}
};
public List<BluetoothDevice> getDeviceList() {
return mDeviceList;
}
}
```
在上面的代码中,我们首先创建了一个 `BleScanner` 类来扫描外围BLE蓝牙设备。`startScan()` 方法接受一个 `serviceUuid` 作为参数,用于筛选特定类型的设备。在此方法中,我们使用 BluetoothLeScanner 开始扫描,并在发现符合条件的设备时连接设备并发现服务。如果符合条件的服务被发现,则将设备添加到设备列表中。之后,我们使用 `stopScan()` 方法停止扫描。
在扫描结束后,您可以使用 `getDeviceList()` 方法获取扫描到的设备列表。请注意,这个列表只包含筛选后符合条件的设备。
希望这个例子对您有所帮助!
阅读全文
相关推荐
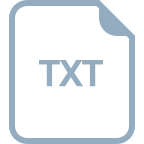


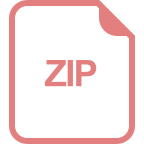
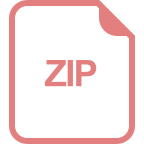
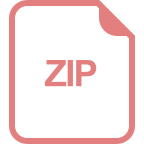
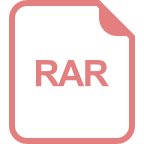
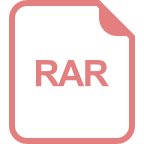
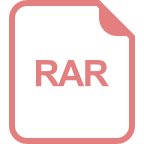
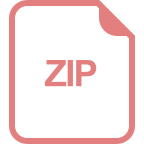
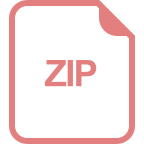
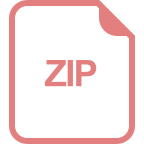
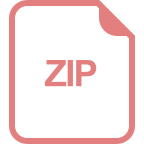
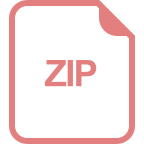
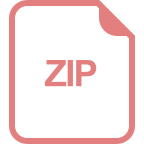
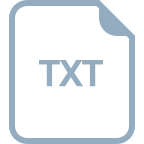
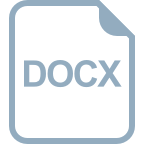