使用8086汇编语言输入一组字符串,统计该字符串中的大写字母、小写字母和数字的个数,并在屏幕上显示
时间: 2023-06-11 08:08:30 浏览: 170
下面是使用8086汇编语言实现统计字符串中大写字母、小写字母和数字的个数的示例代码:
```
.model small
.stack 100h
.data
msg1 db 'Enter a string: $'
msg2 db 'Number of uppercase letters: $'
msg3 db 'Number of lowercase letters: $'
msg4 db 'Number of digits: $'
str_buffer db 80
count_upper dw 0
count_lower dw 0
count_digit dw 0
.code
main proc
mov ax, @data
mov ds, ax
; display message to enter a string
mov ah, 09h
lea dx, msg1
int 21h
; read string from keyboard
mov ah, 0ah
lea dx, str_buffer
int 21h
; count uppercase, lowercase, and digits
mov si, offset str_buffer+1 ; skip over length byte
mov cx, [si] ; get length of string
inc si ; move to first character
mov ah, 0 ; clear high byte of AX
count_loop:
lodsb ; load next character into AL
cmp al, 'A'
jb not_upper
cmp al, 'Z'
ja not_upper
inc count_upper
jmp next_char
not_upper:
cmp al, 'a'
jb not_lower
cmp al, 'z'
ja not_lower
inc count_lower
jmp next_char
not_lower:
cmp al, '0'
jb next_char
cmp al, '9'
ja next_char
inc count_digit
next_char:
loop count_loop
; display results
mov ah, 09h
lea dx, msg2
int 21h
mov ax, count_upper
call print_num
mov ah, 09h
lea dx, msg3
int 21h
mov ax, count_lower
call print_num
mov ah, 09h
lea dx, msg4
int 21h
mov ax, count_digit
call print_num
mov ah, 4ch
int 21h
main endp
; subroutine to print a number in AX
print_num proc
push bx
push cx
push dx
mov bx, 10
xor cx, cx ; CX will be the digit count
digit_loop:
xor dx, dx ; clear DX for DIV instruction
div bx ; divide AX by BX
push dx ; save remainder on stack
inc cx ; increment digit count
test ax, ax ; test if quotient is zero
jnz digit_loop
print_loop:
pop dx ; retrieve digit from stack
add dl, '0' ; convert to ASCII
mov ah, 02h ; BIOS print character function
int 21h
loop print_loop
pop dx
pop cx
pop bx
ret
print_num endp
end main
```
这个程序首先显示提示用户输入字符串的消息,然后使用 `INT 21h` 中断读取用户输入的字符串。它使用一个循环遍历字符串中的每个字符,并使用 `CMP` 指令检查它是否为大写字母、小写字母或数字。如果字符符合其中一个条件,相应的计数器将被递增。最后,程序使用 `INT 21h` 中断打印每个计数器中的值。
注意,这个示例假设用户输入的字符串不超过80个字符。如果需要处理更长的字符串,需要相应地增加缓冲区大小和计数器大小。
阅读全文
相关推荐
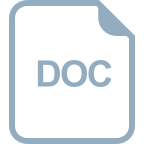
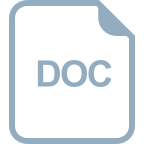
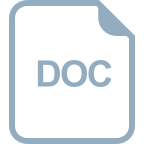

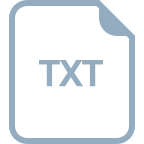













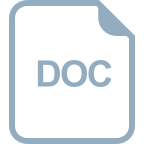