使用链表实现多项式求值
时间: 2023-05-14 19:05:49 浏览: 67
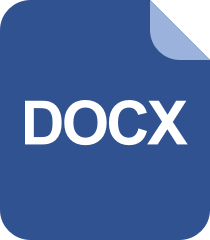
多项式相加链表实现.docx
可以使用链表来实现多项式求值。具体实现方法是,将多项式中的每一项都存储在链表的一个节点中,节点包含两个成员变量:系数和指数。然后遍历链表,将每一项的系数乘以 x 的指数次幂,再将所有项的结果相加即可得到多项式的值。
以下是一个简单的 C++ 代码示例:
```
#include <iostream>
using namespace std;
struct Node {
int coef; // 系数
int exp; // 指数
Node* next;
};
// 遍历链表,计算多项式的值
int evaluate(Node* head, int x) {
int result = 0;
Node* p = head;
while (p != nullptr) {
result += p->coef * pow(x, p->exp);
p = p->next;
}
return result;
}
int main() {
// 创建链表,存储多项式 2x^3 + 3x^2 + 4x + 5
Node* head = new Node{2, 3, new Node{3, 2, new Node{4, 1, new Node{5, 0, nullptr}}}};
int x = 2;
int result = evaluate(head, x);
cout << "The value of the polynomial at x = " << x << " is " << result << endl;
return 0;
}
```
输出结果为:
```
The value of the polynomial at x = 2 is 29
```
阅读全文
相关推荐
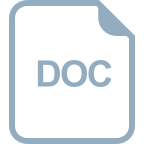
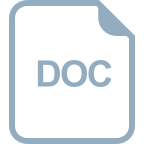





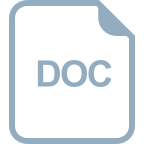
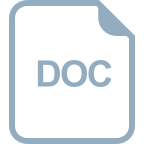
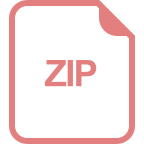
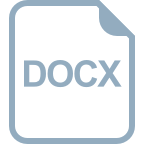