使用python+pyqt+mysql编写的python三层架构项目
时间: 2023-11-23 18:05:55 浏览: 69
Python三层架构是一种常见的软件开发模式,将应用程序分为三个独立的组件:表示层、业务逻辑层和数据访问层。其中,表示层负责呈现用户界面,业务逻辑层负责处理业务逻辑,数据访问层负责与数据库交互。
使用Python+PyQt+MySQL编写的三层架构项目可以实现图形化的用户界面,同时实现对MySQL数据库的连接和数据操作。以下是一个简单的示例代码:
表示层(GUI):
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QLineEdit, QPushButton
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('My App')
self.setGeometry(100, 100, 400, 300)
self.label = QLabel('Enter your name:', self)
self.label.move(50, 50)
self.name_input = QLineEdit(self)
self.name_input.move(150, 50)
self.greet_button = QPushButton('Greet', self)
self.greet_button.move(150, 100)
self.greet_button.clicked.connect(self.greet)
def greet(self):
name = self.name_input.text()
message = f'Hello, {name}!'
self.statusBar().showMessage(message)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
业务逻辑层:
```python
class GreetingService:
def greet(self, name):
return f'Hello, {name}!'
```
数据访问层:
```python
import mysql.connector
class UserRepository:
def __init__(self, host, user, password, database):
self.connection = mysql.connector.connect(
host=host,
user=user,
password=password,
database=database
)
self.cursor = self.connection.cursor()
def get_users(self):
self.cursor.execute('SELECT * FROM users')
return self.cursor.fetchall()
def add_user(self, name, email):
sql = 'INSERT INTO users (name, email) VALUES (%s, %s)'
values = (name, email)
self.cursor.execute(sql, values)
self.connection.commit()
```
在业务逻辑层中,可以调用数据访问层的方法来实现对MySQL数据库的连接和数据操作。例如:
```python
class GreetingService:
def __init__(self, user_repository):
self.user_repository = user_repository
def greet(self, name):
users = self.user_repository.get_users()
message = f'Hello, {name}! There are {len(users)} users in the database.'
return message
```
这样,就可以实现一个简单的Python三层架构项目。
相关推荐
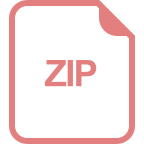
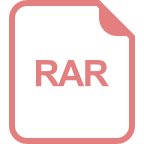
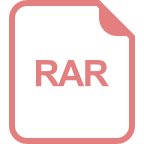
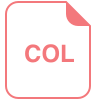
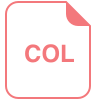
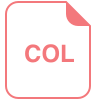
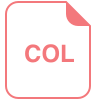










