写一个Java程序,查询excel表中某一列非重复项的个数
时间: 2023-05-28 11:02:17 浏览: 78
以下是一个使用Apache POI库和HashSet数据结构的Java程序,可以查询Excel表中某一列非重复项的数量:
```java
import java.io.FileInputStream;
import java.io.IOException;
import java.util.HashSet;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelColumnDistinctCount {
public static void main(String[] args) throws IOException {
FileInputStream file = new FileInputStream("excel.xlsx"); // 替换为实际文件路径
XSSFWorkbook workbook = new XSSFWorkbook(file); // 创建Excel工作簿对象
Sheet sheet = workbook.getSheetAt(0); // 获取第一个工作表
HashSet<String> distinctValues = new HashSet<>(); // 创建HashSet存储不重复的数据
for (Row row : sheet) {
Cell cell = row.getCell(0); // 读取第一列的单元格数据
if (cell != null && cell.getCellType() != Cell.CELL_TYPE_BLANK) {
String value = cell.getStringCellValue();
if (!distinctValues.contains(value)) {
distinctValues.add(value);
}
}
}
System.out.println("不重复的数据个数为:" + distinctValues.size());
workbook.close(); // 关闭工作簿对象
}
}
```
其中,文件名为 "excel.xlsx" 的Excel文件位于项目的根目录下。程序逐行读取第一列的单元格数据,将非重复的数据存储在HashSet中,最后输出HashSet元素的数量即为不重复数据的个数。
阅读全文
相关推荐
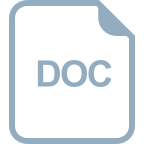
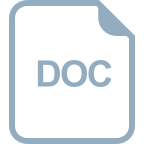
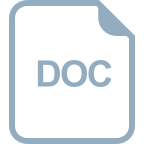
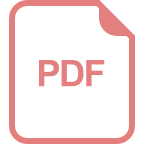
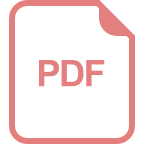
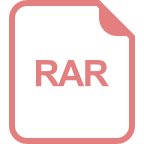
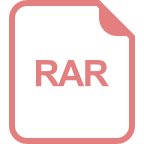
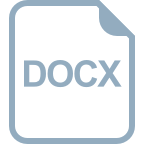
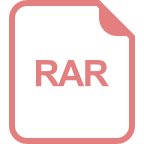
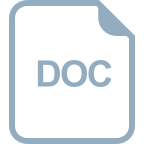
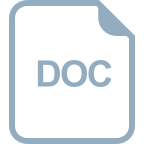
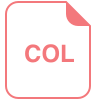
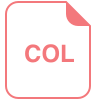
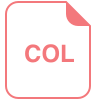
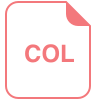
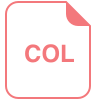
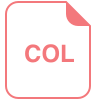
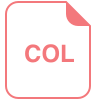
