C语言设计一考勤系统。考勤信息记录了学生的缺课情况,它包括:缺课日期、第几节课、课程名称、学生姓名、学生学号、缺课类型(迟到、早退、请假及旷课)。系统具有以下功能: 1、 录入学生的缺课记录; 2、 修改某个学生的缺课记录; 3、 查询某个学生的缺课情况; 4、 统计某段时间内,旷课学生姓名及旷课次数,按旷课次数由多到少排序; 5、 统计某段时间内,有学生旷课的课程及旷课人次,按旷课人次由多到少排序; 6、 系统以菜单方式工作。
时间: 2023-07-10 20:03:59 浏览: 153
以下是一个基本的考勤系统的C语言实现:
```c
#include <stdio.h>
#include <string.h>
#define MAX_STUDENTS 100
#define MAX_ABSENCES 200
typedef struct {
int day;
int period;
char course[50];
char name[50];
char id[20];
char type[10];
} Absence;
typedef struct {
char name[50];
char id[20];
int num_absences;
Absence absences[MAX_ABSENCES];
} Student;
Student students[MAX_STUDENTS];
int num_students = 0;
void add_absence() {
printf("Enter absence information:\n");
Absence absence;
printf("Day: ");
scanf("%d", &absence.day);
printf("Period: ");
scanf("%d", &absence.period);
printf("Course: ");
scanf("%s", absence.course);
printf("Name: ");
scanf("%s", absence.name);
printf("ID: ");
scanf("%s", absence.id);
printf("Type (late, early, leave, absent): ");
scanf("%s", absence.type);
// Find the student and add the absence to their record
int i;
for (i = 0; i < num_students; i++) {
if (strcmp(students[i].name, absence.name) == 0 && strcmp(students[i].id, absence.id) == 0) {
students[i].absences[students[i].num_absences] = absence;
students[i].num_absences++;
printf("Absence added.\n");
return;
}
}
// If the student wasn't found, create a new record for them
Student student;
strcpy(student.name, absence.name);
strcpy(student.id, absence.id);
student.absences[0] = absence;
student.num_absences = 1;
students[num_students] = student;
num_students++;
printf("Student and absence added.\n");
}
void modify_absence() {
char name[50];
char id[20];
printf("Enter the name and ID of the student whose absence you want to modify:\n");
scanf("%s %s", name, id);
// Find the student
int i, j;
for (i = 0; i < num_students; i++) {
if (strcmp(students[i].name, name) == 0 && strcmp(students[i].id, id) == 0) {
// Display the student's absences and prompt the user to choose one to modify
printf("Student found. Here are their absences:\n");
for (j = 0; j < students[i].num_absences; j++) {
printf("%d %d %s %s %s %s\n", students[i].absences[j].day, students[i].absences[j].period,
students[i].absences[j].course, students[i].absences[j].name, students[i].absences[j].id,
students[i].absences[j].type);
}
printf("Enter the number of the absence you want to modify:\n");
int num;
scanf("%d", &num);
if (num < 0 || num >= students[i].num_absences) {
printf("Invalid absence number.\n");
return;
}
// Modify the absence
Absence absence = students[i].absences[num];
printf("Enter new information for the absence:\n");
printf("Day: ");
scanf("%d", &absence.day);
printf("Period: ");
scanf("%d", &absence.period);
printf("Course: ");
scanf("%s", absence.course);
printf("Name: ");
scanf("%s", absence.name);
printf("ID: ");
scanf("%s", absence.id);
printf("Type (late, early, leave, absent): ");
scanf("%s", absence.type);
students[i].absences[num] = absence;
printf("Absence modified.\n");
return;
}
}
printf("Student not found.\n");
}
void display_student() {
char name[50];
char id[20];
printf("Enter the name and ID of the student whose absences you want to display:\n");
scanf("%s %s", name, id);
// Find the student and display their absences
int i, j;
for (i = 0; i < num_students; i++) {
if (strcmp(students[i].name, name) == 0 && strcmp(students[i].id, id) == 0) {
printf("Student found. Here are their absences:\n");
for (j = 0; j < students[i].num_absences; j++) {
printf("%d %d %s %s %s %s\n", students[i].absences[j].day, students[i].absences[j].period,
students[i].absences[j].course, students[i].absences[j].name, students[i].absences[j].id,
students[i].absences[j].type);
}
return;
}
}
printf("Student not found.\n");
}
void sort_students(int arr[], int n) {
int i, j;
for (i = 0; i < n-1; i++) {
for (j = 0; j < n-i-1; j++) {
if (arr[j] < arr[j+1]) {
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
void display_ranked_students() {
int start_day, end_day;
printf("Enter the starting and ending days of the period you want to check:\n");
scanf("%d %d", &start_day, &end_day);
// Count the number of absences for each student in the period
int num_absences[num_students];
int i, j;
for (i = 0; i < num_students; i++) {
num_absences[i] = 0;
for (j = 0; j < students[i].num_absences; j++) {
if (students[i].absences[j].day >= start_day && students[i].absences[j].day <= end_day
&& strcmp(students[i].absences[j].type, "absent") == 0) {
num_absences[i]++;
}
}
}
// Sort the students by number of absences and display them
sort_students(num_absences, num_students);
printf("Students ranked by number of absences:\n");
for (i = 0; i < num_students; i++) {
for (j = 0; j < num_students; j++) {
if (num_absences[i] == 0) {
break;
}
if (strcmp(students[j].id, "") != 0 && num_absences[i] == students[j].num_absences) {
printf("%s: %d absences\n", students[j].name, num_absences[i]);
students[j].id[0] = '\0'; // Mark the student as already displayed
break;
}
}
}
}
void display_ranked_courses() {
int start_day, end_day;
printf("Enter the starting and ending days of the period you want to check:\n");
scanf("%d %d", &start_day, &end_day);
// Count the number of absences for each course in the period
char courses[MAX_ABSENCES][50];
int num_absences[MAX_ABSENCES];
int num_courses = 0;
int i, j, k;
for (i = 0; i < num_students; i++) {
for (j = 0; j < students[i].num_absences; j++) {
if (students[i].absences[j].day >= start_day && students[i].absences[j].day <= end_day
&& strcmp(students[i].absences[j].type, "absent") == 0) {
// Check if the course has already been added to the array
int found = 0;
for (k = 0; k < num_courses; k++) {
if (strcmp(courses[k], students[i].absences[j].course) == 0) {
num_absences[k]++;
found = 1;
break;
}
}
// If not, add it
if (!found) {
strcpy(courses[num_courses], students[i].absences[j].course);
num_absences[num_courses] = 1;
num_courses++;
}
}
}
}
// Sort the courses by number of absences and display them
sort_students(num_absences, num_courses);
printf("Courses ranked by number of absences:\n");
for (i = 0; i < num_courses; i++) {
for (j = 0; j < num_courses; j++) {
if (num_absences[i] == 0) {
break;
}
if (strcmp(courses[j], "") != 0 && num_absences[i] == num_absences[j]) {
printf("%s: %d absences\n", courses[j], num_absences[i]);
courses[j][0] = '\0'; // Mark the course as already displayed
break;
}
}
}
}
int main() {
int choice;
do {
printf("\nAttendance System Main Menu\n");
printf("1. Add an absence\n");
printf("2. Modify an absence\n");
printf("3. Display a student's absences\n");
printf("4. Display ranked list of students with most absences\n");
printf("5. Display ranked list of courses with most absences\n");
printf("6. Quit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_absence();
break;
case 2:
modify_absence();
break;
case 3:
display_student();
break;
case 4:
display_ranked_students();
break;
case 5:
display_ranked_courses();
break;
case 6:
printf("Goodbye!\n");
break;
default:
printf("Invalid choice.\n");
break;
}
} while (choice != 6);
return 0;
}
```
该程序使用结构体来存储每个学生的考勤记录,并提供了菜单选项来添加、修改、查询和统计缺课记录。`sort_students`函数使用冒泡排序将一个整数数组按降序排列。`display_ranked_students`和`display_ranked_courses`函数分别统计某段时间内旷课最多的学生和课程,并按旷课次数由多到少排序后显示。
阅读全文
相关推荐
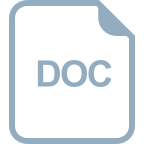
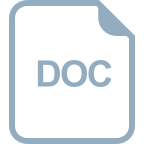
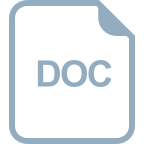

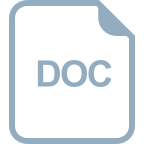
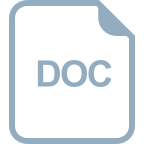
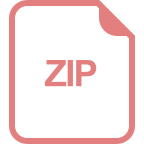




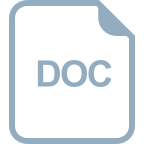
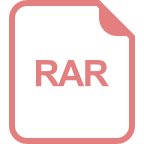
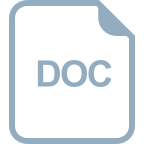
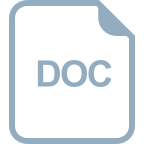
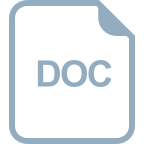
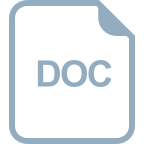

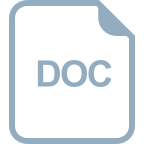