请帮我写一个注册页面的HTML+css+javasript代码,要求完成了输入验证
时间: 2024-01-15 09:04:03 浏览: 68
好的,以下是一个简单的注册页面示例,包含基本的 HTML, CSS 和 JavaScript 代码,用于输入验证:
HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>注册页面</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<h1>注册页面</h1>
<form id="registration-form" action="" method="POST">
<div class="form-control">
<label for="username">用户名:</label>
<input type="text" id="username" name="username" placeholder="请输入用户名" required>
</div>
<div class="form-control">
<label for="email">电子邮件:</label>
<input type="email" id="email" name="email" placeholder="请输入电子邮件" required>
</div>
<div class="form-control">
<label for="password">密码:</label>
<input type="password" id="password" name="password" placeholder="请输入密码" required>
</div>
<div class="form-control">
<label for="confirm-password">确认密码:</label>
<input type="password" id="confirm-password" name="confirm-password" placeholder="请再次输入密码" required>
</div>
<button type="submit" class="btn">注册</button>
</form>
</div>
<script src="script.js"></script>
</body>
</html>
```
CSS代码:
```css
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
}
.container {
max-width: 500px;
margin: 50px auto;
padding: 30px;
background-color: #fff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
h1 {
text-align: center;
margin-bottom: 30px;
}
.form-control {
margin-bottom: 20px;
}
label {
display: block;
margin-bottom: 5px;
font-size: 16px;
font-weight: bold;
}
input[type="text"],
input[type="email"],
input[type="password"] {
width: 100%;
padding: 10px;
border: 1px solid #ccc;
border-radius: 5px;
font-size: 16px;
}
.btn {
display: block;
width: 100%;
padding: 10px;
background-color: #4CAF50;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
font-size: 16px;
}
.btn:hover {
background-color: #3e8e41;
}
```
JavaScript代码:
```javascript
const form = document.getElementById('registration-form');
const username = document.getElementById('username');
const email = document.getElementById('email');
const password = document.getElementById('password');
const confirmPassword = document.getElementById('confirm-password');
form.addEventListener('submit', function(event) {
event.preventDefault();
checkInputs();
});
function checkInputs() {
// 获取输入值并去除空格
const usernameValue = username.value.trim();
const emailValue = email.value.trim();
const passwordValue = password.value.trim();
const confirmPasswordValue = confirmPassword.value.trim();
if (usernameValue === '') {
setErrorFor(username, '用户名不能为空');
} else {
setSuccessFor(username);
}
if (emailValue === '') {
setErrorFor(email, '电子邮件不能为空');
} else if (!isEmail(emailValue)) {
setErrorFor(email, '请输入有效的电子邮件地址');
} else {
setSuccessFor(email);
}
if (passwordValue === '') {
setErrorFor(password, '密码不能为空');
} else {
setSuccessFor(password);
}
if (confirmPasswordValue === '') {
setErrorFor(confirmPassword, '请再次输入密码');
} else if (passwordValue !== confirmPasswordValue) {
setErrorFor(confirmPassword, '两次输入的密码不一致');
} else {
setSuccessFor(confirmPassword);
}
}
function setErrorFor(input, message) {
const formControl = input.parentElement;
const errorMessage = formControl.querySelector('.error-message');
input.classList.add('error');
errorMessage.innerText = message;
formControl.classList.add('error');
}
function setSuccessFor(input) {
const formControl = input.parentElement;
input.classList.remove('error');
formControl.classList.remove('error');
}
function isEmail(email) {
// 正则表达式验证电子邮件格式
const re = /^(([^<>()[\]\\.,;:\s@\"]+(\.[^<>()[\]\\.,;:\s@\"]+)*)|(\".+\"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/;
return re.test(email);
}
```
在这个示例中,我们使用了一些基本的 HTML 元素,如表单、输入框和按钮,并使用 CSS 对它们进行样式化。我们还使用了一些 JavaScript,用于对用户输入进行验证,并在必要时显示错误消息。
在 JavaScript 中,我们定义了一个 `checkInputs()` 函数,该函数将获取用户输入的值,并使用 `setErrorFor()` 和 `setSuccessFor()` 函数进行输入验证。`setErrorFor()` 函数将在输入无效时显示错误消息,而 `setSuccessFor()` 函数将在输入有效时清除错误消息。
最后,我们使用了 `addEventListener()` 方法来监听表单提交事件,并使用 `preventDefault()` 方法防止表单默认提交行为。如果输入验证通过,则表单将提交,否则将显示错误消息。
阅读全文
相关推荐
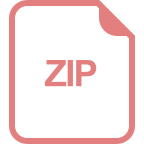
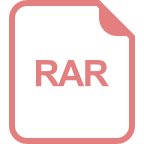
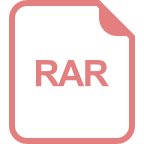
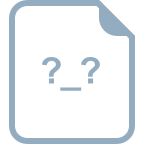
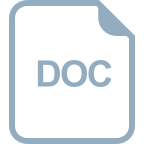
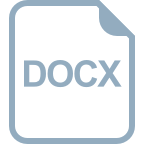
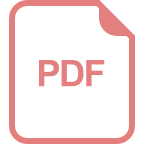
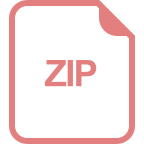
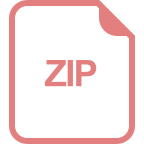
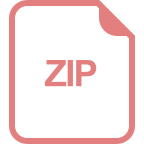
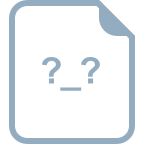
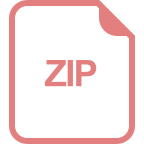
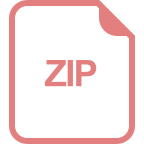
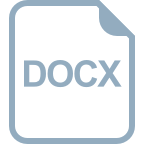
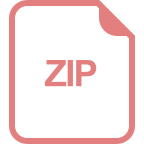
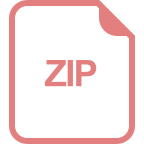