Pyqt5实现界面thrift rpc 连接
时间: 2023-09-26 19:13:42 浏览: 57
PyQt5 是一个 Python 的 GUI 库,Thrift 是一个跨语言的服务框架。在 PyQt5 中实现 Thrift RPC 连接主要需要以下步骤:
1. 安装 thrift 库
在终端中执行以下命令安装 thrift 库:
```
pip install thrift
```
2. 编写 thrift 文件
定义 Thrift 服务的接口和数据类型,将其保存为 .thrift 文件。
例如,定义一个简单的服务接口:
```
service Calculator {
i32 add(1:i32 num1, 2:i32 num2),
i32 sub(1:i32 num1, 2:i32 num2),
}
```
3. 生成 Python 代码
使用 thrift 工具生成 Python 代码。在终端中执行以下命令:
```
thrift -r --gen py calculator.thrift
```
4. 编写 PyQt5 界面
使用 PyQt5 设计界面,添加按钮等控件。
5. 编写 Thrift 客户端
在 PyQt5 代码中编写 Thrift 客户端,调用 Thrift 服务。
例如,实现 Thrift 客户端代码:
```python
from thrift.transport import TSocket
from thrift.transport import TTransport
from thrift.protocol import TBinaryProtocol
from thrift.protocol import TMultiplexedProtocol
from calculator import Calculator
class ThriftClient(object):
def __init__(self, host, port):
self.transport = TSocket.TSocket(host, port)
self.transport = TTransport.TBufferedTransport(self.transport)
protocol = TBinaryProtocol.TBinaryProtocol(self.transport)
self.client = Calculator.Client(TMultiplexedProtocol(protocol, "Calculator"))
def open(self):
self.transport.open()
def close(self):
self.transport.close()
def add(self, num1, num2):
return self.client.add(num1, num2)
def sub(self, num1, num2):
return self.client.sub(num1, num2)
```
6. 连接 Thrift 服务
在 PyQt5 代码中连接 Thrift 服务,调用 Thrift 客户端。
例如,连接 Thrift 服务:
```python
client = ThriftClient("localhost", 9090)
client.open()
result = client.add(1, 2)
client.close()
```
这样就可以在 PyQt5 界面中实现 Thrift RPC 连接了。
相关推荐
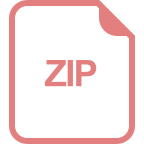
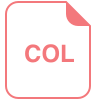
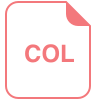
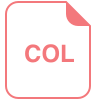
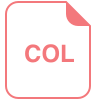
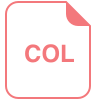









