python中统计字符串个数
时间: 2023-08-24 11:07:21 浏览: 166
你可以使用count()方法来统计一个字符串中某个子字符串出现的次数。比如,如果你想统计字符串s中子字符串sub出现的次数,你可以这样做:
```
s = "hello world"
sub = "l"
count = s.count(sub)
print(count)
```
这将输出字符串s中子字符串"l"出现的次数,即3。如果你想统计字符串s中不同子字符串出现的次数,你可以使用Python中的collections模块中的Counter类来实现。例如:
```
from collections import Counter
s = "hello world"
c = Counter(s)
print(c)
```
这将输出一个Counter对象,其中包含了字符串s中每个字符出现的次数。
相关问题
python统计字符串中个数
在Python中,你可以使用内置函数来统计字符串中特定字符、单词或其他元素的数量。这里有几个常用的例子:
1. **计数字母个数**:
```python
string = "Hello, World!"
count = string.count("o") # 统计字母"o"出现的次数
print(count)
```
2. **计数空格个数**:
```python
text = "这是一个测试文本"
space_count = text.count(" ") # 统计空格的数量
print(space_count)
```
3. **计数特定字符集或子串**:
```python
s = "Python programming"
char_set_count = len(set(s)) # 计算字符集中唯一字符的数量(忽略重复)
substring_count = s.count("in") # 统计子串"in"出现的次数
print(char_set_count, substring_count)
```
4. **使用正则表达式**(如果你需要更复杂模式的匹配):
```python
import re
regex = r'\d+' # 匹配所有连续数字
num_count = len(re.findall(regex, "This is a test with 123 and 456 numbers")) # 统计数字的个数
print(num_count)
```
python统计字符串中字符个数
好的,问题收到。下面是Python统计字符串中字符个数的代码实现:
```python
string = input("请输入字符串:") # 输入字符串
char_count = {} # 创建一个空字典
for char in string: # 遍历字符串中的每个字符
if char in char_count: # 如果字符已经在字典中,计数加1
char_count[char] += 1
else: # 如果字符不在字典中,添加键值对并计数为1
char_count[char] = 1
print("各字符出现次数为:")
for key, value in char_count.items(): # 遍历字典中的所有键值对
print(key, ":", value) # 输出键和对应的值
```
运行程序后,输入一个字符串,程序会输出该字符串中每个字符出现的次数。希望我的回答能够帮助到你!
阅读全文
相关推荐
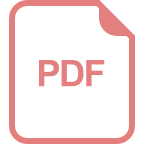
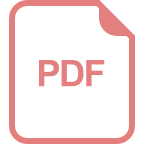
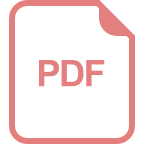












