现在有SH39F325A AFE芯片 和SCH8815 帮我写一段主机控制两个从机的通信I2C通信程序
时间: 2024-03-09 09:46:42 浏览: 166
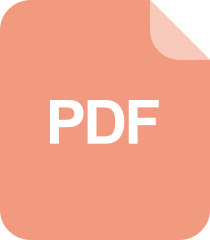
F325 中文使用说明书.pdf
好的,下面是一个简单的示例程序,演示了如何使用I2C主机控制SH39F325A AFE芯片和SCH8815两个从机的通信:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <linux/i2c-dev.h>
#define I2C_BUS "/dev/i2c-1"
#define SH39F325A_ADDR 0x48
#define SCH8815_ADDR 0x50
int main()
{
int fd;
uint8_t sh39f325a_data[] = {0x01, 0x02, 0x03, 0x04};
uint8_t sch8815_data[] = {0x05, 0x06, 0x07, 0x08};
uint8_t sh39f325a_read_data[4];
uint8_t sch8815_read_data[4];
// 打开I2C总线
fd = open(I2C_BUS, O_RDWR);
if (fd < 0) {
perror("Failed to open I2C bus");
exit(1);
}
// 设置SH39F325A从机地址
if (ioctl(fd, I2C_SLAVE, SH39F325A_ADDR) < 0) {
perror("Failed to set SH39F325A I2C slave address");
exit(1);
}
// 写入数据到SH39F325A从机
if (write(fd, sh39f325a_data, sizeof(sh39f325a_data)) != sizeof(sh39f325a_data)) {
perror("Failed to write data to SH39F325A I2C slave");
exit(1);
}
// 读取SH39F325A从机返回的数据
if (read(fd, sh39f325a_read_data, sizeof(sh39f325a_read_data)) != sizeof(sh39f325a_read_data)) {
perror("Failed to read data from SH39F325A I2C slave");
exit(1);
}
// 设置SCH8815从机地址
if (ioctl(fd, I2C_SLAVE, SCH8815_ADDR) < 0) {
perror("Failed to set SCH8815 I2C slave address");
exit(1);
}
// 写入数据到SCH8815从机
if (write(fd, sch8815_data, sizeof(sch8815_data)) != sizeof(sch8815_data)) {
perror("Failed to write data to SCH8815 I2C slave");
exit(1);
}
// 读取SCH8815从机返回的数据
if (read(fd, sch8815_read_data, sizeof(sch8815_read_data)) != sizeof(sch8815_read_data)) {
perror("Failed to read data from SCH8815 I2C slave");
exit(1);
}
// 关闭I2C总线
close(fd);
// 打印读取到的数据
printf("Read data from SH39F325A I2C slave: ");
for (int i = 0; i < sizeof(sh39f325a_read_data); i++) {
printf("%02x ", sh39f325a_read_data[i]);
}
printf("\n");
printf("Read data from SCH8815 I2C slave: ");
for (int i = 0; i < sizeof(sch8815_read_data); i++) {
printf("%02x ", sch8815_read_data[i]);
}
printf("\n");
return 0;
}
```
在上面的示例程序中,我们打开I2C总线并设置SH39F325A AFE芯片从机地址,然后写入数据到SH39F325A从机,并读取SH39F325A从机返回的数据。接着,我们设置SCH8815从机地址,写入数据到SCH8815从机,并读取SCH8815从机返回的数据。最后,我们关闭I2C总线并打印读取到的数据。
需要注意的是,具体的I2C通信协议和数据格式需要参考芯片的数据手册,根据实际需要进行修改。同时,程序中的地址和数据也需要根据实际情况进行修改。
阅读全文
相关推荐
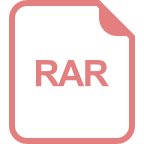
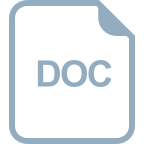

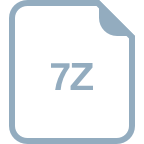
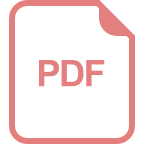
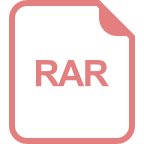
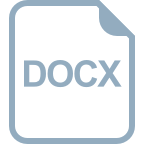
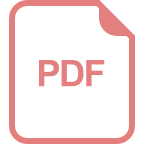
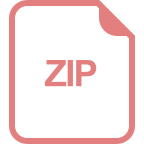
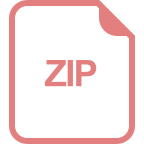
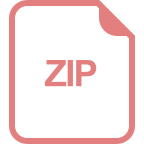
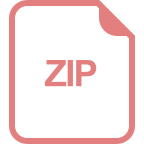
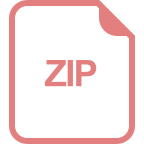
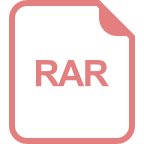
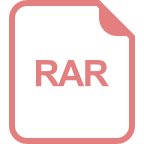