write java。Once you have checked that your methods readLine and readPosInt work correctly, remove all the code inside the main method of the CLI class so that the main method is empty again. In the rest of this question, use the readLine and readPosInt methods every time your program needs to read a string or an integer from the user. In the empty main method of the CLI class, create a single Library object with the name "hhh Library". The main method of the CLI class must then print a menu that allows the user of your system to do six different actions that involve the library object, and your program must then read an integer from the user that indicates which action must be performed by the program (see below for the details about each action). Use the readPosInt method to print the menu (give the string for the menu as the argument of readPosInt) and to read the integer typed by the user.
时间: 2023-07-02 21:04:09 浏览: 63
Here is an example implementation of the CLI class with the required functionality:
```java
import java.util.Scanner;
public class CLI {
private static final String MENU = "1. Add a book\n2. Remove a book\n3. Search for a book\n4. Borrow a book\n5. Return a book\n6. Quit";
private static Library library;
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
library = new Library("hhh Library");
int choice = -1;
while (choice != 6) {
System.out.println(MENU);
choice = readPosInt(scanner, "Enter your choice: ");
switch (choice) {
case 1:
addBook(scanner);
break;
case 2:
removeBook(scanner);
break;
case 3:
searchBook(scanner);
break;
case 4:
borrowBook(scanner);
break;
case 5:
returnBook(scanner);
break;
case 6:
System.out.println("Goodbye!");
break;
default:
System.out.println("Invalid choice. Please try again.");
break;
}
}
}
private static void addBook(Scanner scanner) {
String title = readLine(scanner, "Enter the title of the book: ");
String author = readLine(scanner, "Enter the author of the book: ");
int year = readPosInt(scanner, "Enter the publication year of the book: ");
library.addBook(new Book(title, author, year));
System.out.println("Book added successfully!");
}
private static void removeBook(Scanner scanner) {
String title = readLine(scanner, "Enter the title of the book to remove: ");
boolean removed = library.removeBook(title);
if (removed) {
System.out.println("Book removed successfully!");
} else {
System.out.println("Book not found.");
}
}
private static void searchBook(Scanner scanner) {
String searchTerm = readLine(scanner, "Enter a search term for the title or author of the book: ");
Book[] results = library.searchBooks(searchTerm);
if (results.length == 0) {
System.out.println("No results found.");
} else {
System.out.println("Search results:");
for (Book book : results) {
System.out.println(book);
}
}
}
private static void borrowBook(Scanner scanner) {
String title = readLine(scanner, "Enter the title of the book to borrow: ");
boolean borrowed = library.borrowBook(title);
if (borrowed) {
System.out.println("Book borrowed successfully!");
} else {
System.out.println("Book not available for borrowing.");
}
}
private static void returnBook(Scanner scanner) {
String title = readLine(scanner, "Enter the title of the book to return: ");
boolean returned = library.returnBook(title);
if (returned) {
System.out.println("Book returned successfully!");
} else {
System.out.println("Book not found or not borrowed.");
}
}
private static String readLine(Scanner scanner, String message) {
System.out.print(message);
return scanner.nextLine();
}
private static int readPosInt(Scanner scanner, String message) {
int num = -1;
while (num < 0) {
System.out.print(message);
try {
num = Integer.parseInt(scanner.nextLine());
} catch (NumberFormatException e) {
System.out.println("Invalid input. Please enter a positive integer.");
}
}
return num;
}
}
```
This implementation uses a Scanner object to read input from the user, and provides a menu with six different actions that can be performed on the library object. Each action is implemented as a separate method that reads input from the user as necessary and calls the appropriate method on the library object. The readLine and readPosInt methods are used to read strings and integers from the user, respectively, and handle any validation or error checking that may be required.
相关推荐
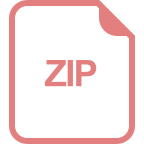
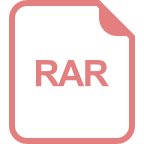















