def get_conv_filter(self, name): return tf.constant(self.data_dict[name][0], name="filter") def get_bias(self, name): return tf.constant(self.data_dict[name][1], name="biases") def get_fc_weight(self, name): return tf.constant(self.data_dict[name][0], name="weights")
时间: 2024-01-20 08:01:56 浏览: 67
这段代码实现了获取预训练模型中某一层的权重参数和偏置参数的功能。其中,get_conv_filter函数获取卷积层的权重参数,get_bias函数获取偏置参数,get_fc_weight函数获取全连接层的权重参数。这些参数都是通过读取预训练模型文件(如VGGNet)中的参数来实现的,通过常量张量的形式返回。
相关问题
class MobileInvertedResidualBlock(BasicUnit): def __init__(self, mobile_inverted_conv, shortcut): super(MobileInvertedResidualBlock, self).__init__() self.mobile_inverted_conv = mobile_inverted_conv self.shortcut = shortcut def forward(self, x): if self.mobile_inverted_conv.is_zero_layer(): res = x elif self.shortcut is None or self.shortcut.is_zero_layer(): res = self.mobile_inverted_conv(x) else: conv_x = self.mobile_inverted_conv(x) skip_x = self.shortcut(x) res = skip_x + conv_x return res @property def unit_str(self): return '(%s, %s)' % (self.mobile_inverted_conv.unit_str, self.shortcut.unit_str if self.shortcut is not None else None) @property def config(self): return { 'name': MobileInvertedResidualBlock.__name__, 'mobile_inverted_conv': self.mobile_inverted_conv.config, 'shortcut': self.shortcut.config if self.shortcut is not None else None, } @staticmethod def build_from_config(config): mobile_inverted_conv = set_layer_from_config( config['mobile_inverted_conv']) shortcut = set_layer_from_config(config['shortcut']) return MobileInvertedResidualBlock(mobile_inverted_conv, shortcut) def get_flops(self, x): flops1, _ = self.mobile_inverted_conv.get_flops(x) if self.shortcut: flops2, _ = self.shortcut.get_flops(x) else: flops2 = 0 return flops1 + flops2, self.forward(x)
这段代码定义了MobileInvertedResidualBlock类,它表示ProxylessNAS中的一个Mobile Inverted Residual Block。Mobile Inverted Residual Block是一种基于MobileNetV2的轻量级神经网络模块,用于构建ProxylessNAS网络架构。
MobileInvertedResidualBlock类的构造函数接受两个参数:mobile_inverted_conv和shortcut。mobile_inverted_conv是Mobile Inverted Convolution的实例,用于实现卷积操作;shortcut是一个可选项,用于实现跳跃连接。MobileInvertedResidualBlock类的前向函数forward(x)接受输入张量x,并根据是否存在shortcut来计算输出张量res。
MobileInvertedResidualBlock类还有unit_str属性和config属性,用于获取表示该类的字符串和配置字典。build_from_config方法根据配置字典构造一个MobileInvertedResidualBlock对象。get_flops方法用于获取MobileInvertedResidualBlock的计算代价(FLOPS)。
def avg_pool(self, bottom, name): return tf.nn.avg_pool(bottom, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME', name=name) def max_pool(self, bottom, name): return tf.nn.max_pool(bottom, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME', name=name) def conv_layer(self, bottom, name): with tf.variable_scope(name): filt = self.get_conv_filter(name) conv = tf.nn.conv2d(bottom, filt, [1, 1, 1, 1], padding='SAME') conv_biases = self.get_bias(name) bias = tf.nn.bias_add(conv, conv_biases) relu = tf.nn.relu(bias) return relu
这段代码定义了三个函数,用于构建VGG19模型中的卷积层和池化层。
avg_pool()函数用于定义平均池化层,其中bottom表示输入tensor,ksize表示池化窗口大小为[1, 2, 2, 1],strides表示步长为[1, 2, 2, 1],padding表示填充方式为'SAME'。
max_pool()函数用于定义最大池化层,其输入参数和参数设置与avg_pool()函数类似,只不过池化的方式不同。
conv_layer()函数用于定义卷积层,其中bottom表示输入tensor,name表示该卷积层的名称。在函数内部,首先调用了get_conv_filter()函数获取该卷积层的卷积核,然后使用tf.nn.conv2d()函数进行卷积操作,再调用get_bias()函数获取该卷积层的偏置,使用tf.nn.bias_add()函数加上偏置,最后使用ReLU激活函数激活输出结果,并将其返回。
阅读全文
相关推荐
















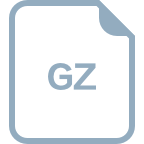